In this article we will look at different types of inheritance and what types of inheritance are supported in Java.
There are five types of inheritance based on how many base classes and derived classes are there in the inheritance relationship. They are as follows:
- Simple inheritance
- Multi-level inheritance
- Multiple inheritance
- Hierarchical inheritance
- Hybrid inheritance
Let’s look at each one of these five types of inheritance in more detail.
Contents
Simple inheritance
This is the most frequently used and most simple of the five types of inheritance. In this type of inheritance there is a single derived class which inherits from a single base class. This can be represented pictorially as shown below:
In the above figure, A is base class and B is derived class. This can be converted to Java code as given below:
class A
{
//Members of class A
}
class B extends A
{
//Members of class B
}
Multi-level Inheritance
In this type of inheritance there are several classes in the hierarchy forming multiple levels. Each level contains a base class and a derived class. Multi-level inheritance can be represented as shown below:
In the above figure there are two levels. First level contains A as the base class and B as the derived class. In the second level B is the base class and C is the derived class. The above figure can be converted to Java code as given below:
class A
{
//Members of class A
}
class B extends A
{
//Members of class B
}
class C extends B
{
//Members of class C
}
Multiple Inheritance
In this type of inheritance a single derived class can inherit from two or more base classes. Multiple inheritance can be represented as shown below:
In the above figure A and B are base classes and C is the derived class. Above figure can be converted to Java code as given below:
class A
{
//Members of class A
}
class B
{
//Members of class B
}
class C extends A,B
{
//Members of class C
}
It is important to remember that in Java, we cannot inherit from more than one class. So in the above code class C extends A, B is syntactically incorrect.
Hierarchical Inheritance
In this type of inheritance, two or more derived classes inherit from a common base class. Hierarchical inheritance can be represented as shown below:
In the above figure A is the base class and B, C and D are derived classes. Above figure can be converted to Java code as given below:
class A
{
//Members of class A
}
class B extends A
{
//Members of class B
}
class C extends A
{
//Members of class C
}
class D extends A
{
//Members of class D
}
Hybrid Inheritance
As the name itself implies, hybrid inheritance is a combination of any two or more of the above mentioned four types of inheritance. Hybrid inheritance can be represented as shown below:
In the above figure you can see hierarchical, single and multi-level inheritance. Above figure can be converted to Java code as given below:
class A
{
//Members of class A
}
class B extends A
{
//Members of class B
}
class C extends A
{
//Members of class C
}
class D extends A
{
//Members of class D
}
class E extends B
{
//Members of class E
}
Note: It is not mandatory to write the classes in a particular order in Java. You can write in any order that you want.
What types of inheritance does Java support?
Java supports all types of inheritance mentioned above except multiple inheritance. Java doesn’t allow multiple inheritance in case of classes but it allows multiple inheritance in case of interfaces. As interfaces are not yet introduced, I will cover this in future articles.
Why multiple inheritance (among classes) has been removed in Java? Well, there are certain problems in multiple inheritance. For example, consider that a derived class C inherits from classes A and B (multiple inheritance). Suppose there are is a variable by the name X in both classes A and B. Now, if I access X in class C (which is valid due to inheritance) where does X value come from. Does it come from A or B?
Above problem is also valid for methods and not only for variables. Such situations leads to ambiguity. To eliminate such ambiguous situations, Java designers voted for removing multiple inheritance in the case of classes.
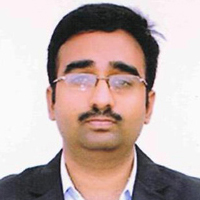
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply