In this article you will learn about Java expressions. You will look at what is an expression in Java, what factors are considered while evaluating Java expressions and some examples which will make you clear on expressions in Java.
Contents
Introduction
An expression is a construct which is made up of literals, variables, method calls and operators following the syntax of Java. Every expressions consists of at least one operator and an operand. Operand can be either a literal, variable or a method invocation.
Following are some of the examples for expressions in Java:
How expressions are evaluated?
It is common for an expression to have more than one operator. For example, consider the below example:
(20 * 5) + (10 / 2) – (3 * 10)
So, how is the above expression evaluated? Expression evaluation in Java is based upon the following concepts:
- Type promotion rules
- Operator precedence
- Associativity rules
I have already explained the type promotion rules here. Since all are integer values, there is no need to worry about type promotion rules here. We will now concentrate on operator precedence and associativity rules.
Operator precedence
All the operators in Java are divided into several groups and are assigned a precedence level. The operator precedence chart for the operators in Java is shown below:
Now let’s consider the following expression:
10 – 2 * 5
One will evaluate the above expression normally as, 10-2 gives 8 and then 8*5 gives 40. But Java evaluates the above expression differently. Based on the operator precedence chart shown above, * has higher precedence than +. So, 2* 5 is evaluated first which gives 10 and then 10 – 10 is evaluated which gives 0.
What if the expression contains two or more operators from the same group? Such ambiguities are solved using the associativity rules.
Associativity Rules
When an expression contains operators from the same group, associativity rules are applied to determine which operation should be performed first. The associativity rules of Java are shown below:
Now, let’s consider the following expression:
10-6+2
In the above expression, the operators + and – both belong to the same group in the operator precedence chart. So, we have to check the associativity rules for evaluating the above expression. Associativity rule for + and – group is left-to-right i.e, evaluate the expression from left to right. So, 10-6 is evaluated to 4 and then 4+2 is evaluated to 6.
Use of parentheses in expressions
Let’s look at our original expression example:
(20 * 5) + (10 / 2) – (3 * 10)
You might think that, what is the need of parenthesis ( and ) in the above expression. The reason I had included them is, parenthesis have the highest priority (precedence) over all the operators in Java.
So, in the above expression, (20*5) is evaluated to 100, (10/2) is evaluated to 5 and (3*10) is evaluated to 30. Now, our intermediate expression looks like:
100 + 5 – 30
Now, we can apply the associativity rules and evaluate the expression. The final answer for the above expression is 75.
There is another popular use of parenthesis. We will use them in print statements. For example consider the following piece of code:
int a=10, b=20;
System.out.println(“Sum of a and b is: “+a+b);
One might think that the above code will produce the output: “Sum of a and b is: 30”. The real output will be:
Sum of a and b is: 1020
Why? Because, when one of the operand inside a print statement is a string, the + operator acts as a concatenation operator. To make it behave as an arithmetic operator we should enclose a+b is parenthesis as shown below:
int a=10, b=20;
System.out.println(“Sum of a and b is: “+(a+b));
Now (a+b) is evaluated first and then concatenated to the rest.
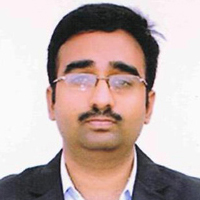
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
bookmarked!!, I love your site!