In this article we will look at final keyword in Java. Also we will see what is the use of final keyword in inheritance along with Java code samples.
Uses of final Keyword
Following are the uses of final keyword in Java:
- To declare constant values
- To make a method non-overridable
- To make a class non-inheritable
The second and third uses of final are applicable only in the context of inheritance. Let’s look at each of the uses of final keyword in detail.
Declaring constant values
The first use of final is to declare constant values in Java programs. Syntax for declaring a constant is shown below:
final data-type variable-name = value;
An example for declaring constants is given below:
final float PI = 3.1415;
In Java, you have to assign the value for the constant in the declaration time itself.
final for methods
The final keyword can be used to make a method in base class non-overridable. Syntax for making a method non-overridable is given below:
final return-type method-name(parameters-list)
{
//Body of the method
}
So, we can say that an abstract method cannot be declared as final.
final for classes
The final keyword can be used to make a class non-inheritable or non-extendable. Syntax for making a class non-inheritable is given below:
final class Class-Name
{
//Members of class
}
So, we can say that an abstract class cannot be declared as final.
Let’s consider an example to demonstrate the use of final keyword in inheritance:
abstract class Shape
{
final void area()
{
System.out.println("Area of shape");
}
}
class Circle extends Shape
{
void area()
{
System.out.println("Area of circle");
}
}
class Rectangle extends Shape
{
void area()
{
System.out.println("Area of rectangle");
}
}
public class Driver
{
public static void main(String[] args)
{
Shape s;
s = new Circle();
s.area();
s = new Rectangle();
s.area();
}
}
The above code when compiled gives errors as the sub classes are trying to override the area() method in the super class which was declared as final.
Now, let’s look at another example:
final class Shape
{
void area()
{
System.out.println("Area of shape");
}
}
class Circle extends Shape
{
void area()
{
System.out.println("Area of circle");
}
}
class Rectangle extends Shape
{
void area()
{
System.out.println("Area of rectangle");
}
}
public class Driver
{
public static void main(String[] args)
{
Shape s;
s = new Circle();
s.area();
s = new Rectangle();
s.area();
}
}
Above code gives errors because the classes Circle and Rectangle are trying to inherit the Shape class which was declared final (non-inheritable).
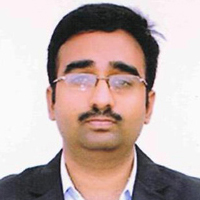
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply