In this article you will learn about the scope and lifetime of variables in a Java program. Different types of variables in Java have different scope and lifetime.
Introduction
Scope of a variable refers to in which areas or sections of a program can the variable be accessed and lifetime of a variable refers to how long the variable stays alive in memory.
General convention for a variable’s scope is, it is accessible only within the block in which it is declared. A block begins with a left curly brace { and ends with a right curly brace }.
As we know there are three types of variables: 1) instance variables, 2) class variables and 3) local variables, we will look at the scope and lifetime of each of them now.
Instance Variables
A variable which is declared inside a class and outside all the methods and blocks is an instance variable.
General scope of an instance variable is throughout the class except in static methods. Lifetime of an instance variable is until the object stays in memory.
Class Variables
A variable which is declared inside a class, outside all the blocks and is marked static is known as a class variable.
General scope of a class variable is throughout the class and the lifetime of a class variable is until the end of the program or as long as the class is loaded in memory.
Local Variables
All other variables which are not instance and class variables are treated as local variables including the parameters in a method.
Scope of a local variable is within the block in which it is declared and the lifetime of a local variable is until the control leaves the block in which it is declared.
Nested Scope
In Java, we can create nested blocks – a block inside another block. In case of nested blocks what is the scope of local variables?
All the local variables in the outer block are accessible within the inner block but vice versa is not true i.e., local variables within the inner block are not accessible in the outer block. Consider the following example:
class Sample
{
public static void main(String[] args)
{
int x;
//Begining of inner block
{
int y = 100;
x = 200;
System.out.println("x = "+x);
}
//End of inner block
System.out.println("x = "+x);
y = 200; //Error as y is not accessible in the outer block
}
}
As you can see in the above program, line 14 generates an error as the variable y is not visible in the outer block and therefore cannot be accessed.
The summary of scope and lifetime of variables is as shown below:
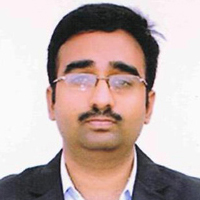
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Thankyou for the important point
Thanks for brief information.
Ya it is very useful …