In this article we will look at what is an exception?, what is exception handling?, and how Java supports exception handling.
Contents
Introduction
In general, the errors in programs can be categorized into three types:
- Syntax errors: Also called compile time errors when a program violates the rules of the programming language. Ex: missing a semi colon, typing a spelling mistake in keyword etc.
- Run-time errors: Errors which are raised during execution of the program due to violation of semantic rules or other abnormal behaviour. Ex: dividing a number with zero, stack overflow, illegal type conversion, accessing an unavailable array element etc.
- Logic errors: Errors which are raised during the execution of the program due to mistakes in the logic applied in the program. These are the most severe kind of errors to detect. Ex: misplacing a minus sign in the place of plus sign, placing a semi colon at the end of loops etc.
Exception: An abnormal condition that occurs at run-time or a run-time error is known as an exception.
Exception Handling: Handling exceptions is known as exception handling. Instead of letting the program crash (terminate abnormally) when an error occurs at run-time, we provide meaningful error messages to the users by handling the exception(s).
Exception Handling Fundamentals
In Java, abnormal conditions that occur in programs are known as exceptions. Java exceptions are objects which are thrown when an exception raises. Exceptions which are raised must be handled (caught) at some point. Exceptions can be created by Java run-time system or they can be created manually.
Java aids exception handling by providing five keywords: try, catch, throw, throws, and finally. Their use is described as follows:
try: Statements that are supposed to raise exception(s) are placed inside a try block.
catch: Code that handles exceptions are placed in catch blocks. A try block must be followed by one or more catch blocks or a single finally block.
throw: Most of the exceptions are thrown automatically by the Java run-time system. We can throw exceptions manually using throw keyword.
throws: If a method which raises exceptions doesn’t want to handle them, they can be thrown to parent method or the Java run-time using the throws keyword.
finally: All the statements that should execute irrespective of whether a exception arises or not is placed in the finally block.
The general form of an exception handling block looks as follows:
try
{
//Statements that might arise exceptions
}
catch(ExceptionType1 obj)
{
//Exception handling code
}
catch(ExceptionType2 obj)
{
//Exception handling code
}
//...
finally
{
//Code that execute even if exception(s) occur or not
}
Note that every try block must be followed by one or more catch blocks or a single finally block. To aid exception handling, Java provides several pre-defined exception classes. The root class for all exception classes is Throwable. The hierarchy of exception classes is as follows:
Exception Types
There are different categorizations of exceptions based on factors given below:
- Nature of exceptions
- Necessity of handling exceptions
- Creation of exceptions
Nature of Exceptions:
Based on nature of exceptions, exceptions are categorized into Exceptions and Errors. Two direct sub classes of the Throwable class are Exception and Error.
All the sub classes of Exception class are related to exceptions raised in the programmer’s code. One important sub class is RuntimeException which contains several sub classes representing exceptions that are automatically handled by the Java run-time system. Examples of such exceptions are divide by zero, array index out of bounds.
All the sub classes of Error class are related to exceptions related to problems with run-time system. Examples of such errors are stack overflow and out of memory.
Necessity of Handling Exceptions:
Based on whether it is compulsory to provide exception handling code or not, exceptions are categorized into checked exceptions and unchecked exceptions.
Exceptions for which providing exception handling is not necessary are known as unchecked exceptions. All sub classes of RuntimeException class are examples of unchecked exceptions.
Exceptions which must be handled by the code written by programmer are known as checked exceptions. Examples of checked exceptions are IOException, ClassNotFoundException etc.
Creation of Exceptions
Based on who is providing the exception classes, exceptions are categorized into pre-defined exceptions (system exceptions) and user-defined exceptions.
All exception classes available with Java are known as pre-defined exceptions or system exceptions. Exceptions that created by the programmer are known as user-defined exceptions.
Exception Handling Example
Let’s see a sample Java program which handles “divide by zero” exception. First let’s see the Java program without using exception handling constructs. The program is given below:
class Divide
{
public static void main(String[] args)
{
int a = 10, b = 0;
int c = a / b;
System.out.println("Result is: " + c);
}
}
The output of the above program is:
Exception in thread “main” java.lang.ArithmeticException: / by zero
at Divide.main(Divide.java:6)
The exception which was automatically generated by Java run-time system was ArithmeticException which is a sub class of RuntimeException. So, all unchecked exceptions like ArithmeticException are automatically handled by Java run-time system.
The above output says that the exception was raised in main method, exception raised was ArithmeticException and the associated message is / by zero, exception was raised in Divide class, in Divide.java file and on line number 6.
Now, let’s use exception handling constructs to provide our own exception handling code (message). The program is given below:
class Divide
{
public static void main(String[] args)
{
try
{
int a = 10, b = 0;
int c = a / b;
System.out.println("Result is: " + c);
}
catch(ArithmeticException e)
{
System.out.println("b cannot be zero");
}
}
}
Output of above program is:
b cannot be zero
This is how we can use exception handling constructs to handle exceptions in Java.
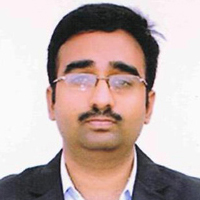
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply