This article explains about the parameter passing techniques in programming languages in general and how Java handles parameters in methods. Sample code is also provided which demonstrates the parameter passing techniques.
Introduction
If you have any previous programming experience you might know that most of the popular programming languages support two parameter passing techniques namely: pass-by-value and pass-by-reference.
In pass-by-value technique, the actual parameters in the method call are copied to the dummy parameters in the method definition. So, whatever changes are performed on the dummy parameters, they are not reflected on the actual parameters as the changes you make are done to the copies and to the originals.
In pass-by-reference technique, reference (address) of the actual parameters are passed to the dummy parameters in the method definition. So, whatever changes are performed on the dummy parameters, they are reflected on the actual parameters too as both references point to same memory locations containing the original values.
To make the concept more simple, let’s consider the following code segment which demonstrates pass-by-value. This is a program for exchanging values in two variables:
class Swapper
{
int a;
int b;
Swapper(int x, int y)
{
a = x;
b = y;
}
void swap(int x, int y)
{
int temp;
temp = x;
x = y;
y = temp;
}
}
class SwapDemo
{
public static void main(String[] args)
{
Swapper obj = new Swapper(10, 20);
System.out.println("Before swapping value of a is "+obj.a+" value of b is "+obj.b);
obj.swap(obj.a, obj.b);
System.out.println("After swapping value of a is "+obj.a+" value of b is "+obj.b);
}
}
Output of the above programming will be:
Before swapping value of a is 10 value of b is 20
After swapping value of a is 10 value of b is 20
Although values of x and y are interchanged, those changes are not reflected on a and b. Memory representation of variables is shown in below figure:
Let’s consider the following code segment which demonstrates pass-by-reference. This is a program for exchanging values in two variables:
class Swapper
{
int a;
int b;
Swapper(int x, int y)
{
a = x;
b = y;
}
void swap(Swapper ref)
{
int temp;
temp = ref.a;
ref.a = ref.b;
ref.b = temp;
}
}
class SwapDemo
{
public static void main(String[] args)
{
Swapper obj = new Swapper(10, 20);
System.out.println("Before swapping value of a is "+obj.a+" value of b is "+obj.b);
obj.swap(obj);
System.out.println("After swapping value of a is "+obj.a+" value of b is "+obj.b);
}
}
Output of the above programming will be:
Before swapping value of a is 10 value of b is 20
After swapping value of a is 20 value of b is 10
The changes performed inside the method swap are reflected on a and b as we have passed the reference obj into ref which also points to the same memory locations as obj. Memory representation of variables is shown in below figure:
Note: In Java, parameters of primitive types are passed by value which is same as pass-by-value and parameters of reference types are also passed by value (the reference is copied) which is same as pass-by-reference. So, in Java all parameters are passed by value only.
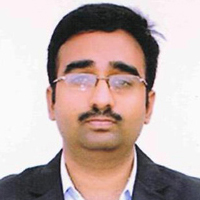
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Thank you soooo much easy and clearly understood