In this article we will learn what are applets in Java, what are the differences between a Java program and an applet and how to create applets.
Contents
Definition of Applet
An applet is a small Java program that is a part of a web page and is downloaded automatically from a server when the client requests that web page.
Applet Fundamentals
Since applet is a part of a webpage, we will learn basic things related to web pages. A web page is a collection of information (ex: Google home page, Yahoo home page etc). Web pages are generally created using HTML.
A web page contains at least four basic HTML tags:
- <html> : Specifies that it is the root tag.
- <head> : Specifies the head section of the web page. It contains meta information, page title, scripts, external style sheet links etc.
- <body> : Contains the content that is displayed to a user who is visiting the web page.
- <title> : Specifies the title of the web page. It is specified inside <head> tags.
As an example let’s look at the HTML code of a web page that displays “Hello World” in large letters. The code is as follows:
hello.html
<html>
<head>
<title>My Webpage</title>
</head>
<body>
<h1>Hello World</h1>
</body>
</html>
The file will be saved with .html extension. When you open the file with a web browser, the output will be as follows:
An applet can be displayed in a web page using the <applet> tag as shown below:
<applet code=”ClassName” height=”200″ width=”400″></applet>
An applet doesn’t contain a main() method like the Java console programs. An applet is used to display graphics or to display a GUI (ex: login form, registration form) to the users.
Creating an Applet
An applet can be created by extending either Applet class or JApplet class. First let’s see how to create an applet using the Applet class. The Applet class is available in java.applet package. An applet which displays “Hello World” is shown below:
MyApplet.java
import java.awt.*;
import java.applet.*;
public class MyApplet extends Applet
{
public void paint(Graphics g)
{
g.drawString("Hello World!", 20, 20);
}
}
/*
<applet code="MyApplet" height="300" width="500"></applet>
*/
Remember to include the <applet> tag in comments. This is useful for running the applet. The value of code attribute must match with the class name.
In the above applet program, the class MyApplet extends the Applet class and it contains a method named paint() which accepts a parameter of the type Graphics. The Graphics class belongs to java.awt package and is used to display text or graphics on our applet.
The paint() method is used to re-display the output when the applet is resized or minimized or maximized. The drawString() method belongs to Graphics class and it displays “Hello World!” on the applet display area at the point whose coordinates are (20, 20).
Save the file as MyApplet.java and compile it to generate MyApplet.class file.
Running an Applet
An applet can be executed in two ways:
- Using appletviewer command-line tool or
- Using a browser
Using appletviewer tool:
Java provides a command line tool named appletviewer for quick debugging of applets. Use the following syntax for running an applet using appletviewer:
appletviewer filename
As the file name of our applet is MyApplet.java, the command for executing the applet is:
appletviewer MyApplet.java
Output of the above command will be as shown below:
Using a browser:
Create a web page named hello.html with the following HTML code:
<html>
<head>
<title>My Webpage</title>
</head>
<body>
<applet code="MyApplet" height="200" width="400"></applet>
</body>
</html>
Unfortunately from Java 7 onwards, local applets (unsigned) cannot be viewed using a browser and Google Chrome browser no longer supports applets in web pages.
It is recommended to use appletviewer tool to debug and execute applets.
Watch below video to learn how to execute Java applets using appletviewer command line tool:
Differences between a Java Application and Applets
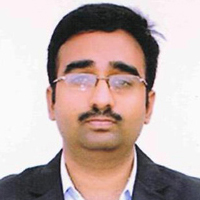
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
This is really useful, thanks.
Sir,how to execute this applet (where to run the command ,
appletviewer MyApplet.java).
You have to run the command in command prompt (cmd) in Windows.
sir, even if the comment section isn’t provided ,it shows the same result.
I am using netbeans IDE , and I am running it in that IDE with the appletviewer.
By command prompt it shows the following error
‘appletviewer’ is not recognized as an internal or external command,
operable program or batch file.
Please make a video on it and send it to me.
arshadbashag@outlook.com
If command prompt says appletviewer is not recongnized, you have to add jdk bin directory to the path environment variable. I will make a video today night or tomorrow. Visit this page again later. I will update the video link here.