In this article we will look at access control in Java. We will learn about four access modifiers: public, protected, default and private along with java code examples.
Introduction
Access control is a mechanism, an attribute of encapsulation which restricts the access of certain members of a class to specific parts of a program. Access to members of a class can be controlled using the access modifiers. There are four access modifiers in Java. They are:
- public
- protected
- default
- private
If the member (variable or method) is not marked as either public or protected or private, the access modifier for that member will be default. We can apply access modifiers to classes also.
Among the four access modifiers, private is the most restrictive access modifier and public is the least restrictive access modifier. Syntax for declaring a access modifier is shown below:
access-modifier data-type variable-name;
Example for declaring a private integer variable is shown below:
private int side;
In a similar way we can apply access modifiers to methods or classes although private classes are less common.
Note: Packages and inheritance will be discussed in another article in future. So, I will defer the explanation of protected to packages article as protected is useful only when there is inheritance.
Accessibility restrictions of the four access modifiers is as shown below:
What is the use of access modifiers?
As I had said above, access modifiers are used to restrict the access of members of a class, in particular data members (fields). Let me explain this through Java code. Let’s consider an employee class as shown below:
class Employee
{
int empid;
String empname;
float salary;
//Methods which operate on above data members
}
By looking at the above code we can say that the access modifier for all the three data members is default. As members with default (also known as Package Private or no modifier) access modifier are accessible throughout the package (in other classes), a programmer might, by mistake, try to make an employee’s salary negative as shown below:
Employee e1 = new Employee();
e1.salary = -1000.00;
Although above code is syntactically correct, it is logically incorrect. To prevent such things to happen, in general, all the data members are declared private and are accessible only through public methods. So, we can modify our Employee class as shown below:
class Employee
{
private int empid;
private String emp;
private float salary;
public void setSalary(float sal)
{
if(sal < 0)
{
System.out.println("Salary cannot be negative");
}
else
{
salary = sal;
}
}
//Other methods
}
By looking at the above code, we can say that one can access the salary field only through setSalary() method. Now, we can set the salary of an employee as shown below:
Employee e1 = new Employee();
e1.setSalary(-1000.00); //Gives error as salary cannot be negative
e1.setSalary(2000.00); //salary of e1 will be assigned 2000.00
The modified Employee class is the best way of writing programs in Java.
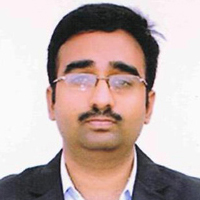
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Yes! Finally someone writes about design.
@srikanth, the if condition is checking if the salary (sal) is less than 0 or not. If it is less than 0, it will print the message inside if block. Otherwise, the value in sal variable will be assigned to salary instance variable.
In the above program & l t ; refers to less than (<) symbol.
can please explain the “if” condition in above stated program.