In this article we will look at method overriding which is similar to method overloading. Method overriding is a mechanism which supports polymorphism in Java.
What is method overriding?
In the context of inheritance, suppose a base class A contains a method display with zero parameters and sub class B also contains a method display with zero parameters, what happens when we create an object for class B and call the display method using that object?
The method that will execute is the display method in the sub class B. Then what happened to the display method in super class A? It was hidden. This process of sub class method hiding the super class method when both methods contains same method signature is known as method overriding.
When is method overriding possible?
Method overriding is possible only when the following things are satisfied:
- A class inherits from another class (inheritance).
- Both super class and sub class should contain a method with same signature.
What is the difference between method overloading and method overriding?
Both may look similar but they are quite different in the following ways:
- Method overloading takes place when a class contains multiple methods with the same name but varying number of parameters or types of parameters.
- Method overriding takes place when two or more classes contains a method with same signature and all of them are participating in inheritance.
Now let’s look at some examples which demonstrates method overriding.
Example 1:
class A
{
public void display()
{
System.out.println("This is display method of class A");
}
}
class B extends A
{
public void display()
{
System.out.println("This is display method of class B");
}
}
public class Driver
{
public static void main(String[] args)
{
B obj = new B();
obj.display();
}
}
In the above example the method display in both classes A and B have same method signature (method name + number of parameters). So, the display method in class B hides the display method in class A. Output of the above program is:
This is display method of class B
Now let’s look at another example.
Example 2:
class A
{
public void display()
{
System.out.println("This is display method of class A");
}
}
class B extends A
{
public void display()
{
System.out.println("This is display method of class B");
}
}
public class Driver
{
public static void main(String[] args)
{
A obj = new A();
obj.display();
}
}
In the above example, even though there are methods with the same signature, as the object obj belongs to class A, the display method of class A is executed and the output is:
This is display method of class A
Now let’s consider another example.
Example 3:
class A
{
public void display()
{
System.out.println("This is display method of class A");
}
}
class B extends A
{
public void display(int x)
{
System.out.println("This is display method of class B");
}
}
public class Driver
{
public static void main(String[] args)
{
B obj = new B();
obj.display();
}
}
In the above example, notice that the display method in the sub class accepts a single integer parameter. Now when we call display method as obj.display() in the Driver class, method overloading takes place and display method of class A is executed. Output of the above program is:
This is display method of class A
Now let’s consider another example.
Example 4:
class A
{
public static void display()
{
System.out.println("This is display method of class A");
}
}
class B extends A
{
public void display()
{
System.out.println("This is display method of class B");
}
}
public class Driver
{
public static void main(String[] args)
{
B obj = new B();
obj.display();
}
}
In the above example, the display method in the super class A has been declared as a static. You have to remember that static methods cannot be overridden. So, the above program gives a compile time error.
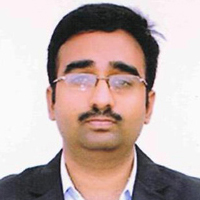
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply