In this article we will look at constructors in Java. We will learn what is a constructor, use of a constructor in Java programs, characteristics of a constructor and different types of a constructor.
Constructor
A constructor is a special method which has the same name as class name and that is used to initialize the objects (fields of an object) of a class. A constructor has the following characteristics:
- Constructor has the same name as the class in which it is defined.
- Constructor doesn’t have a return type, not even void. Implicit return type for a constructor is the class name.
- Constructor is generally used to initialize the objects of a class.
Syntax for creating a constructor is as shown below:
ClassName( [Parameters List] )
{
//Code of the constructor
}
Use of Constructors
A constructor is used for initializing (assigning values to fields) the objects of a class. Can’t we use normal methods for initializing the fields of an object? Why do we require constructors? To make it clear what is the need for constructors, let’s consider an example of where we want to develop a Tic-Tac-Toe game as shown below:
To make it simple, let’s not consider how to display graphics. We will consider only the general details. If you look at the above picture, we have to display a canvas or board (for example) which contains 3×3 = 9 squares. To display the canvas with 9 squares, let’s consider the following class definition:
class TicTacToe
{
Square block1, block2, … , block9;
//Other fields
void display()
{
block1 = new Square();
block1.createBlock();
//Code for rest of the 8 blocks, block2, block3, … , block9
//Code to arrange the blocks as a 3x3 matrix
}
//Other code
}
The Square class will be something as shown below:
class Square
{
int side;
void createBlock()
{
side = 4;
//Code for creating square
}
}
The driver program (which executes our logic classes) will be as shown below:
class Game
{
public static void main(String[] args)
{
TicTacToe board = new TicTacToe();
board.display();
//Other code
}
}
If you look at the above program, the task of display() method is to create 9 square blocks by internally calling createBlock() method of Square class. This whole process can be seen as initializing the board. Every time, when someone wants to play the game, the board has to be initialized (square blocks have to be created and displayed to the user).
To make the initialization process simple, constructors have been introduced whose sole purpose is to initialize the object. Now, let’s modify our previous classes to include constructors. The code is shown below:
class TicTacToe
{
Square block1, block2, … , block9;
//Other fields
TicTacToe()
{
block1 = new Square();
//Code for rest of the 8 blocks, block2, block3, … , block9
//Code to arrange the blocks as a 3x3 matrix
}
//Other code
}
The Square class will be as shown below:
class Square
{
int side;
Square()
{
side = 4;
//Code for creating square
}
}
The driver program (which executes our logic classes) will be as shown below:
class Game
{
public static void main(String[] args)
{
TicTacToe board = new TicTacToe();
//Other code
}
}
If you look at the above code, the methods display() and createBlock() are gone. Remember that initialization might not be only simply assigning values to the fields of an object.
The purpose of a constructor is to initialize the objects such that they will be ready to be used in our program like the board object in above program.
Constructor Invocation
A constructor is invoked (called) automatically whenever an object is created using the new keyword. For example, in the above example, new TicTacToe() calls the constructor of TicTacToe class. If no constructor has been defined, Java automatically invokes the default constructor which initializes all the fields to their default values.
Types of Constructor
Based on the number of parameters and type of parameters, constructors are of three types:
- Parameter less constructor or zero parameter constructor
- Parameterized constructor
- Copy constructor
Parameter less constructor: As the name implies a zero parameter constructor or parameter less constructor doesn’t have any parameters in its signature. These are the most frequently found constructors in a Java program. Let’s consider an example for zero parameter constructor:
class Square
{
int side;
Square()
{
side = 4;
}
}
In the above example, Sqaure() is a zero parameter or parameter less constructor which initializes the side of a square to 4.
Parameterized constructor: This type of constructor contains one or more parameters in its signature. The parameters receive their values when the constructor is called. Let’s consider an example for parameterized constructor:
class Sqaure
{
int side;
Square(int s)
{
side = s;
}
}
In the above example, the Square() constructor accepts a single parameter s, which is used to initialize the side of a square.
Copy constructor: A copy constructor contains atleast one parameter of reference type. This type of constructor is generally used to create copies of the existing objects. Let’s consider an example for copy constructor:
class Sqaure
{
int side;
Square()
{
side = 4;
}
Square(Square original) //This is a copy constructor
{
side = original.side;
}
}
Now let’s an example which covers all three types of constructors:
class Square
{
int side;
Square()
{
side = 4;
}
Square(int s)
{
side = s;
}
Square(Square original)
{
side = original.side;
}
}
The driver program is shown below:
class SquareDemo
{
public static void main(String[] args)
{
Sqaure normal = new Sqaure(); //Invokes zero parameter constructor
Square original = new Sqaure(8); //Invokes single parameter constructor
Sqaure duplicate = new Square(original); //Invokes copy constructor
System.out.println(“Side of normal square is: “+normal.side);
System.out.println(“Side of original square is: “+original.side);
System.out.println(“Side of duplicate square is: “+duplicate.side);
}
}
Run the above program and observe the output.
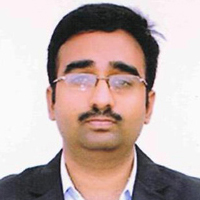
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Way cool! Some extremely valid points! I appreciate you writing this
write-up and also the rest of the website is really good.
This is the right blog for anybody who really wants to understand this topic Wonderful points you have mentioned here,. Its really a great and useful piece of information. Im glad that you shared this helpful information with us.
Please stay us up to date like this. Thanks for sharing.