In this article you are going to learn about overloading in Java. You will look at what is overloading? why should we use overloading? along with details and examples on method overloading and constructor overloading.
Introduction
One of the way through which Java supports polymorphism is overloading. It can be defined as creating two or more methods in the same class sharing a common name but different number of parameters or different types of parameters.
You should remember that overloading doesn’t depend upon the return type of the method. Since method binding is resolved at compile-time based on the number of parameters or type of parameters, overloading is also called as compile-time polymorphism or static binding or early binding.
Why overloading?
In Java, overloading provides the ability to define two or more methods with the same name. What is the use of that? For example, you want to define two methods, in which, one method adds two integers and the second method adds two floating point numbers.
If there is no overloading, we have to create two different methods. One for adding two integers and the other for adding two floating point numbers. As the underlying purpose of the methods is same, why create methods with different name? Instead of creating two different methods, overloading allows us to define two methods with the same name.
Method Overloading
Creating two or more methods in the same class with same name but different number of parameters or different types of parameters is known as method overloading. Let’s consider the following code segment which demonstrates method overloading:
class Addition
{
void sum(int a, int b)
{
System.out.println("Sum of two integers is: "+(a+b));
}
void sum(float a, float b)
{
System.out.println("Sum of two floats is: "+(a+b));
}
}
In the above code segment, the method sum is overloaded. Java compiler decides which method to call based on the type of the parameters in the method call. For example, if the method is called as shown below:
Addition obj = new Addition();
obj.sum(10, 20);
The sum method with two integer parameters will be invoked and the output will be Sum of two integers is: 30.
If the method is called as shown below:
Addition obj = new Addition();
obj.sum(1.5, 1.2);
The sum method with two float parameters will be invoked and the output will be Sum of two floats is: 2.7.
Note: It should be remembered that Java automatically performs type conversion from one type to another type. So, proper care should be taken while defining overloaded methods.
Constructor Overloading
As constructor is a special type of method, constructor can also be overloaded. Several constructors can be defined in the same class given that the parameters vary in each constructor. As an example for constructor overloading, let’s consider the following code segment:
class Square
{
int side;
Square(int s)
{
side = s;
//Code to create a square and return it
}
Sqaure(int s, int n)
{
side = s;
//Code to create n number of squares
}
}
In the above code segment we can see that the constructor Sqaure() is overloaded. One constructor accepts a single integer parameter and returns a single square with side s. Another constructor accepts two integer parameters and returns n number of squares each with side s.
Note: In the above examples I have defined only two overloaded methods or constructors. You can create any number of overloaded methods or constructors based on your requirements.
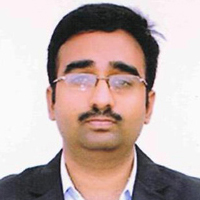
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply