In this article we look at different ways for reading data from a text file in Java.
There are different ways for reading data from a text file which can be used for different types of applications. The multiple ways for reading data from a text file include using BufferedReader, FileReader, Scanner, etc.
Each way for reading provides different features like while reading text using BufferedReader, it provides buffering capability which allows the program to read text faster. While using Scanner, the program can get the ability to parse the text.
Using BufferedReader Class
This way of reading text from a file reads it as character input stream. It can buffer the text for efficient reading of lines, arrays, and characters. The buffer size can be left as default or can be changed base on our need.
In general, the read requests made by FileReader and InputStreamReader make a request to the underlying character and byte stream. So, it is better if those requests can be wrapped using BufferedReader as shown below:
Let’s see a program which uses BufferedReader class for reading and displaying the text in a file. In the below example, the text file name is “sample.txt”.
import java.io.*;
class Driver
{
public static void main(String[] args) throws Exception
{
File f = new File("sample.txt");
BufferedReader br = new BufferedReader(new FileReader(f));
String line;
while((line=br.readLine()) != null)
{
System.out.println(line);
}
}
}
The output of the above program is:
This is a sample text file.
We are learning how to read text from a file.
This is Java fifth unit.
Thank You!
Using FileReader Class
This way provides convenience for reading the text character by character or for reading character files. The constructors of FileReader class are as follows:
- FileReader(File file): Creates a FileReader object based on the file given
- FileReader(FileDescriptor fd): Creates a FileReader object based on the given file descriptor
- FileReader(String filename): Creates a FileReader object based on the given filename
Let’s see a program which uses FileReader class for reading and displaying the text in a file. In the below example, the text file name is “sample.txt”.
import java.io.*;
class Driver
{
public static void main(String[] args) throws Exception
{
FileReader fr = new FileReader("sample.txt");
int c;
while((c=fr.read()) != -1)
{
System.out.print((char)c);
}
}
}
Output of the above program is:
This is a sample text file.
We are learning how to read text from a file.
This is Java fifth unit.
Thank You!
Using Scanner Class
By using Scanner class we can parse the text into primitive data types and strings using regular expressions. A Scanner object can divide the text using a delimiter pattern. By default, the delimiter pattern is white space. After delimiting the text the resulting tokens can be converted into different data types using various next methods.
Let’s see a program which uses Scanner class along with a loop for reading and displaying the text in a file. In the below example, the text file name is “sample.txt”.
import java.io.File;
import java.util.Scanner;
class Driver
{
public static void main(String[] args) throws Exception
{
File f = new File("sample.txt");
Scanner s = new Scanner(f);
while(s.hasNextLine())
{
System.out.println(s.nextLine());
}
}
}
Output of the above program is:
This is a sample text file.
We are learning how to read text from a file.
This is Java fifth unit.
Thank You!
Let’s see a program which uses Scanner class without a loop for reading and displaying the text in a file. In the below example, the text file name is “sample.txt”.
import java.io.File;
import java.util.Scanner;
class Driver
{
public static void main(String[] args) throws Exception
{
File f = new File("sample.txt");
Scanner s = new Scanner(f);
s.useDelimiter(" ");
System.out.println(s.next());
System.out.println(s.next());
System.out.println(s.next());
System.out.println(s.next());
System.out.println(s.next());
}
}
Output of the above program is:
This
is
a
sample
text
For more information, visit the following links:
- https://www.javatpoint.com/java-bufferedreader-class
- https://www.javatpoint.com/java-filereader-class
- https://www.javatpoint.com/Scanner-class
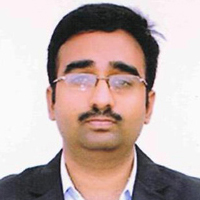
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply