In this article we will learn about inner classes and how can they be used to simplify event handling process along with sample Java programs.
An inner class is a class which is defined inside another class. The inner class can access all the members of an outer class, but vice-versa is not true. The mouse event handling program which was simplified using adapter classes can further be simplified using inner classes.
Following is a Java program which handles the mouse click event using inner class and adapter class:
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class MyApplet extends JApplet
{
JLabel label;
public void init()
{
setSize(600,300);
setLayout(new FlowLayout());
label = new JLabel();
add(label);
addMouseListener(new MyAdapter());
}
class MyAdapter extends MouseAdapter
{
public void mouseClicked(MouseEvent me)
{
label.setText("Mouse is clicked");
}
}
}
In the above example, the class MyAdapter is the inner class which has been declared inside the outer class MyApplet.
Anonymous Inner Classes
Anonymous inner classes are nameless inner classes which can be declared inside another class or as an expression inside a method definition.
The above mouse event handling program can further be simplified by using an anonymous inner class as shown below:
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class MyApplet extends JApplet
{
JLabel label;
public void init()
{
setSize(600,300);
setLayout(new FlowLayout());
label = new JLabel();
add(label);
addMouseListener(new MouseAdapter()
{
public void mouseClicked(MouseEvent me)
{
label.setText("Mouse is clicked");
}
}
);
}
}
In the above program, the syntax new MouseAdapter() {…} says to the compiler that the code written in between the braces represents an anonymous inner class and it extends the class MouseAdapter.
Whenever the line addMouseListener… executes, Java run-time system automatically creates the instance of anonymous inner class.
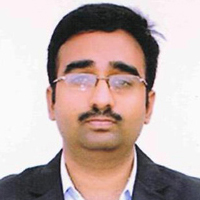
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply