In this article we will learn what is event handling and how to handle events in Java programs using the delegation event model.
Definition: The process of handling events is known as event handling.
Java uses “delegation event model” to process the events raised in a GUI program.
Contents
Delegation Event Model
The delegation event model provides a consistent way of generating and processing events. In this model a source generates an event and sends it to one or more listeners. A listener waits until it receives an event and once it receives an event, processes the event and returns.
The advantage of this model is the application logic for processing the events is clearly separated from the user interface logic. In this model the listeners must register with a source to receive the events.
Another advantage of this model is, notification of events is sent only to the registered listeners.
Events
An event is an object that describes a state change in the source. Some examples of activities that cause events to be generated are clicking a button, pressing a key on the keyboard, selecting an item in the list.
Event Sources
A source is an object that generates an event. A source might generate more than one event. Examples of sources are button, check box, radio button, text box etc.
A source must be registered with listeners in order for the events to be detected. The general form of a registration method is as follows:
public void addTypeListener(TypeListener tl)
A listener can also be unregistered from the source using the remove method whose syntax is as follows:
public void removeTypeListener(TypeListener tl)
Event Listeners
A listener is an object that is notified when an event occurs. A listener has two major requirements. First is, listener must be registered with a source to receive notifications and second is, it must implement methods to receive and process these notifications.
In Java, events and sources are maintained as classes, listeners are maintained as interfaces. Most of them are available in java.awt.event package.
Examples of listeners are MouseListener, Key Listener etc.
java.awt.event Package
The java.awt.event package contains many event classes which can be used for event handling.
Root class for all the event classes in Java is EventObject which is available in java.util package.
Root class for all AWT event classes is AWTEvent which is available in java.awt package and is a sub class of EventObject class.
Some of the frequently used event classes available in java.awt.event package are listed below:
Various constructors and methods available in the above listed event classes are as follows:
ActionEvent
An action event is generated when a button is clicked, or a list item is double-clicked, or a menu item is selected.
ActionEvent class contains the following methods:
String getActionCommand() – Used to obtain the command name for the invoking ActionEvent object.
int getModifiers() – This method returns an integer that indicates which modifier keys (ALT, CTRL, META, and/or SHIFT) were pressed.
long getWhen() – Returns the time when the event was generated.
AdjustmentEvent
The adjustment event is generated when the scroll bar is adjusted. There are five types of adjustment events. For each type there is a constant declared in the class which are as follows:
BLOCK_DECREMENT
BLOCK_INCREMENT
TRACK
UNIT_DECREMENT
UNIT_INCREMENT
Following are the methods available in AdjustmentEvent class:
Adjustable getAdjustable() – Returns the object that generated the event.
int getAdjustmentType() – Returns one of the five constants that represents the type of event.
int getValue() – Returns a number that represents the amount of adjustment.
ComponentEvent
A component event is generated when the visibility, position and size of a component is changed. There are four types of component events which are identified by the following constants:
COMPONENT_HIDDEN
COMPONENT_SHOWN
COMPONENT_MOVED
COMPONENT_RESIZED
Following method is available in the ComponentEvent class:
Component getComponent() – Returns the component that generated the event.
ContainerEvent
A container event is generated when a component is added to or removed from the container. There are two types of container events which are represented by the following constants:
COMPONENT_ADDED
COMPONENT_REMOVED
Following are the methods available in the ContainerEvent class:
Container getContainer() – Returns the reference of the container that generated the event.
Component getChild() – Returns the reference of the component that is added to or removed from the container.
FocusEvent
A focus event is generated when a component gains or loses input focus. These events are identified by the following constants:
FOCUS_GAINED
FOCUS_LOST
Following are the methods available in the FocusEvent class:
Component getOppositeComponent() – Returns the other component that gained or lost focus.
boolean isTemporary() – Method returns true or false that indicates whether the change in focus is temporary or not.
ItemEvent
An item event is generated when the user clicks on a check box or clicks a list item or selects / deselects a checkable menu item. These events are identified by the following constants:
SELECTED
DESELECTED
Following are the methods available in the ItemEvent class:
Object getItem() – Returns a reference to the item whose state has changed.
ItemSelectable getItemSelectable() – Returns the reference of ItemSelectable object that raised the event.
int getStateChange() – Returns the status of the state (whether SELECTED or DESELECTED)
KeyEvent
A key event is generated when a key on the keyboard is pressed, released or typed. These events are identified by the following constants:
KEY_PRESSED (when a key is pressed)
KEY_RELEASED (when a key is released)
KEY_TYPED (when a character is typed)
There are several other constants which recognizes various keys on the keyboard and they are as follows:
KeyEvent is a sub class of InputEvent. Following are the methods available in KeyEvent class:
char getKeyChar() – Returns the character entered from the keyboard
int getKeyCode() – Returns the key code
MouseEvent
The mouse event is generated when the user generates a mouse event on a source. Mouse events are represented by the following constants:
Following are some of the methods available in MouseEvent class:
int getX() – Returns the x-coordinate of the mouse at which the event was generated.
int getY() – Returns the y-coordinate of the mouse at which the event was generated.
Point getPoint() – Returns the x and y coordinates of mouse at which the event was generated.
int getClickCount() – This method returns the number of mouse clicks for an event.
int getButton() – Returns an integer that represents the buttons that caused the event. Returned value can be either NOBUTTON, BUTTON1 (left mouse button), BUTTON2 (middle mouse button), or BUTTON3 (right mouse button).
Following methods are available to obtain the coordinates relative to the screen:
Point getLocationOnScreen()
int getXOnScreen()
int getYOnScreen()
TextEvent
The text event is generated when the user enters a character into a text field or a text area. This event is identified by the following constant:
TEXT_VALUE_CHANGED
WindowEvent
A window event is generated when a user performs any one of the window related events which are identified by the following constants:
Following are some of the methods available in the WindowEvent class:
Window getWindow() – Returns the reference of the window on which the event is generated.
Window getOppositeWindow() – Returns the reference to the previous window when a focus event of activation event has occurred.
int getOldState() – Returns an integer indicating the old state of the window.
int getNewState() – Returns an integer indicating the new state of the window.
Sources of Events
All GUI elements which are derived from the Component class are examples of sources which generates events. Following are some of the examples of event sources available in Java:
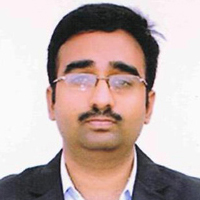
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply