In this article we will learn about built in objects in JavaScript like Array, Date, Math, String and Number and the methods in them.
Introduction
To solve different kinds of problems, JavaScript provides various built-in objects. Each object contains properties and methods. Some of the built-in objects in Javascript are:
- Array
- Date
- Math
- String
- Number
Array object
The properties available on Array object are:
Property | Description |
length | Returns the number of elements in the array |
constructor | Returns the function that created the array object |
prototype | Allows us to add properties and methods to an array object |
Methods available on Array object are:
Method | Description |
reverse() | Reverses the array elements |
concat() | Joins two or more arrays |
sort() | Sort the elements of an array |
push() | Appends one or more elements at the end of an array |
pop() | Removes and returns the last element |
shift() | Removes and returns the first element |
unshift() | Adds one or more elements at the beginning of an array |
join() | Combines the elements of an array by a specified separator |
indexOf() | Returns the first index of specified element |
lastIndexOf() | Returns the last index of specified element |
slice(startindex, endindex) | Returns selected elements |
Math object
The Math object provides a number of properties and methods to work with Number values. Among the methods there are sin and cos for trigonometric functions, floor and round for truncating and rounding the given numbers and max for returning the maximum of given numbers.
Properties available on Math object are:
Property | Description |
PI | The value of Pi |
E | The base of natural logarithm e |
LN2 | Natural logarithm of 2 |
LN10 | Natural logarithm of 10 |
LOG2E | Base 2 logarithm of e |
LOG10E | Base 10 logarithm of e |
SQRT2 | Square root of 2 |
SQRT1_2 | Square root of 1/2 |
Methods available on Math object are:
Method | Description |
max(a,b) | Returns largest of a and b |
min(a,b) | Returns least of a and b |
round(a) | Returns nearest integer |
ceil(a) | Rounds up. Returns the smallest integer greater than or equal to a |
floor(a) | Rounds down. Returns the largest integer smaller than or equal to a |
exp(a) | Returns ea |
pow(a,b) | Returns ab |
abs(a) | Returns absolute value of a |
random() | Returns a pseudo random number between 0 and 1 |
sqrt(a) | Returns square root of a |
sin(a) | Returns sin of a (a is in radians) |
cos(a) | Returns cos of a (a is in radians) |
Date object
At times you there will be a need to access the current date and time and also past and future date and times. JavaScript provides support for working with dates and time through the Date object.
Date objected is created using the new operator and one of the Date’s constructors. Current date and time can be retrieved as shown below:
var today = new Date( );
Methods available on Date object are:
Method | Description |
Date() | Creates a Date object with the current date and time of the browser’s PC |
Date(“Month,dd, yyyy hh:mm:ss”) | This creates a Date object with the specified string |
Date(“Month dd, yyyy”) | This creates a Date object with the specified string |
Date(yy,mm,dd,hh,mm,ss) | This creates a Date object with the specified string |
Date(yy,mm,dd) | This creates a Date object with the specified date |
Date(milliseconds) | Creates a Date object with the date value represented by the number of milliseconds since midnight on Jan 1, 1970 |
getFullYear() | Returns the 4-digit year component of the date. |
getMonth() | Returns the month component of the date. |
getDate() | Returns the date component of the date. |
getDay() | Returns the day component of the date |
getHours() | Returns the hours component of the date |
getMinutes() | Retrieves the minutes component of the date |
getSeconds() | Retrieves the seconds component of the date |
getMilliseconds() | Returns the milliseconds component of the date |
getTimezoneOffset() | Retrieves the difference, in minutes, between the computer’s local time and GMT |
setFullYear() | Sets the year component of the date using a 4-digit number |
setMonth() | Sets the month component of the date |
setDate() | Sets the day-of-month component of the date |
setHours() | Set the hours component of the date |
setMinutes | Set the minutes component of the date |
setSeconds() | Sets the seconds component of the date |
setMilliseconds() | Sets the milliseconds component of the date |
String object
A string is a collection of characters. Most of the times in scripts, there is a need to work with strings. JavaScript provides various properties and methods to work with String objects. Whenever a String property or method is used on a string value, it will be coerced to a String object.
One most frequently used property on String objects is length. The length property gives the number of characters in the given string. Consider the following example:
var str = “Hello World”;
var len = str.length;
The value stored in the len variable will be 11 which the number of characters in the string.
Methods available on String objects are:
Method | Description |
anchor() | Create a HTML anchor element around the specified string |
big() | Create a HTML big element around the specified string |
charAt(index) | Returns the character at specified index |
charCodeAt(index) | Returns the unicode value of the character at specified index |
concat() | Combines two or more strings |
indexOf(searchtext, index) | Searches for the specified string from the beginning of the string. |
lastIndexof(searchtext, index) | Searches for the specified string from the end of the string |
match(expr) | Searches for a specified string in the given string and returns an array of matched strings |
replace(expr, new-string) | Replaces the old string with the specified new string |
search(expr) | Searches for the string/regular expression and returns its index |
slice(start, end) | Extracts the sub string between the specified indices |
split(delimiter, count) | Split the string into an array of substrings delimited by the delimiter |
substr(start, length) | Returns a substring having length characters from a specified index |
toUpperCase() | Returns the string after converting all characters to upper case |
toLowerCase() | Returns the string after converting all characters to lower case |
Number Object
The number object contains a collection of useful properties and methods to work with Numbers. The properties include: MAX_VALUE (represents largest number that is available), MIN_VALUE (represents smallest number that is available), NaN (represents Not a Number), POSITIVE_INFINITY (special value to represent infinity), NEGATIVE_INFINITY (special value to represent negative infinity) and PI (represents value of π.
To test whether the value in a variable is NaN or not, there is a method isNaN( var ), which returns true if the value in the specified variable is NaN and false otherwise. To convert a Number to a String, use the method toString( ) as shown below:
var cost = 250;
var output = cost.toString( );
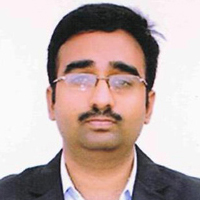
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply