A session is a collection of HTTP requests, over a period of time, between a client and server. Maintaining the data within the session is known as session tracking. For example, maintaining the book details added to the cart in an online book shop application. Session tracking mechanisms include the following:
- URL rewriting (query strings)
- Hidden form fields
- Cookies
- HTTP session (Session objects)
URL Rewriting
In this method we keep track of the data by passing the data as a query string from one page to another page. A query string starts with ? symbol and is followed by set of key-value pairs. Each pair is separated using the & symbol. Passing huge amount of data using query string is cumbersome. This method is recommended only when the data that is needed to be passed is very less. Following example demonstrates passing data using query string:
index.html
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Homepage</title>
</head>
<body>
<a href="ServletA?uname=teja&pass=1234">Go to ServletA</a>
</body>
</html>
ServletA.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class ServletA
*/
public class ServletA extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public ServletA() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.getWriter().print("Welcome: "+request.getParameter("uname")+" your password is: "+request.getParameter("pass"));
response.getWriter().print("<br/>");
response.getWriter().print("<a href='ServletB?uname=teja&pass=1234'>Go to ServletB</a>");
}
}
ServletB.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class ServletB
*/
public class ServletB extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public ServletB() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.getWriter().print("Welcome: "+request.getParameter("uname")+" your password is: "+request.getParameter("pass"));
}
}
In the above example we are passing the data uname and pass from index.html to ServletA and from ServletA to ServletB using query string.
Video: URL Rewriting
Hidden Form Fields
Another way of passing data from one page to another page is by using a hidden form field. The advantage with this method is data is not visible to the user directly. But, when the user looks at the source of the web page in a browser, the data being passed will be visible. Following example demonstrates hidden form fields:
index.html
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Hidden Fields</title>
</head>
<body>
<form action="ServletA" method="post">
<input type="hidden" value="admin" name="username" />
<input type="hidden" value="pass" name="password" />
<input type="submit" value="Go to Servlet" />
</form>
</body>
</html>
ServletA.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class ServletA
*/
public class ServletA extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public ServletA() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String un = request.getParameter("username");
String pw = request.getParameter("password");
response.getWriter().print("Welcome "+un+" your password is: "+pw);
}
}
In the above example two hidden form fields were used to pass data to the servlet file.
Video: Hidden Fields
Cookies
A cookie is a file containing the information sent by the web server to the client. Cookies are stored on client machine. A cookie consists of various attributes such as name, value, message, domain, path, comment and version number. Cookies should only be used to store non-sensitive information.
The servlet API provides a class Cookie available in the javax.servlet.http package which provides a way to manage cookies in web applications. To send a cookie to the client, use addCookie(Cookie c) method of the HttpServletResponse object. To gather the cookies on the client side, use getCookies() method of HttpServletRequest object.
To create a cookie, we can use the constructor of the Cookie class as shown below:
Cookie c = new Cookie(name, value);
Cookie class provides the following methods:
- setValue(String s)
- getValue()
- getName()
- setComment(String s)
- getComment()
- setVersion(String s), getVersion()
- setDomain(String s), getDomain()
- setPath(String s), getPath()
- setSecure(boolean), getSecure(boolean)
Following are the advantages of cookies:
- Cookies reduce network traffic when compared to URL rewriting.
- Cookies maintain data on client side.
- Cookies simplifies the application logic when compared to query strings.
Following are the disadvantages of cookies:
- Cookies are not secure.
- Cookies are HTTP specific.
- Cookies size is limited (4KB in general).
- Cookies can be disabled on client side.
Following example demonstrates storing and retrieving cookies:
cookies.html
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Create Cookies</title>
</head>
<body>
<form action="AddCookies" method="get">
<input type="submit" value="Create Cookies!" />
</form>
</body>
</html>
AddCookies.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class AddCookies
*/
public class AddCookies extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public AddCookies() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Cookie c1 = new Cookie("user1", "pass1");
Cookie c2 = new Cookie("user2", "pass2");
Cookie c3 = new Cookie("user3", "pass3");
Cookie c4 = new Cookie("user4", "pass4");
response.addCookie(c1);
response.addCookie(c2);
response.addCookie(c3);
response.addCookie(c4);
response.getWriter().print("Cookies created successfully!<br/>");
response.getWriter().print("<a href='login.html'>Go to login page</a>");
}
}
login.html
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Login</title>
</head>
<body>
<form action="ValidServ" method="post">
Username: <input type="text" name="txtuser" /><br/>
Password: <input type="password" name="txtpass" /><br/>
<input type="submit" value="Submit" />
<input type="reset" value="clear" />
</form>
</body>
</html>
ValidServ.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class ValidServ
*/
public class ValidServ extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public ValidServ() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String un = request.getParameter("txtuser");
String pw = request.getParameter("txtpass");
boolean flag = false;
Cookie c[] = request.getCookies();
for(int i = 0; i < c.length; i++)
{
if(c[i].getName().equals(un) && c[i].getValue().equals(pw))
{
flag = true;
}
}
if(flag)
response.getWriter().print("Valid User!");
else
response.getWriter().print("Invalid User!");
}
}
Video: Cookies
Session Object
Session object allows the user to store session data on the server-side. This may be a burden on the server if the application is accessed by large number of users. Servlet API provides HttpSession interface to manage the session objects.
We can get the reference of a session object by calling getSession() of HttpServletRequest as shown below:
HttpSession session = request.getSession();
HttpSession interface provides the following functionality:
- Object getAttribute(String name)
- Enumeration getAttributeNames()
- String getId()
- void invalidate()
- void setAttribute(String name, Object value)
- void removeAttribute(String name)
Following example demonstrates adding and retrieving data from a session object:
login.html
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Login</title>
</head>
<body>
<form action="createSession" method="get">
Username: <input type="text" name="txtuser" /><br />
Password: <input type="password" name="txtpass" /><br />
<input type="submit" value="Submit" />
<input type="reset" value="Clear" />
</form>
</body>
</html>
createSession.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
/**
* Servlet implementation class createSession
*/
public class createSession extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public createSession() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String un = request.getParameter("txtuser");
String pw = request.getParameter("txtpass");
if(un.equals("vishnu") && pw.equals("college"))
{
HttpSession session = request.getSession();
session.setAttribute("uname", un);
session.setAttribute("pass", pw);
response.getWriter().print("Valid User!<br/>");
response.getWriter().print("<a href='userHome'>Go to User Home</a>");
}
else
{
response.getWriter().print("Bad username or password");
}
}
}
userHome.java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
/**
* Servlet implementation class userHome
*/
public class userHome extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public userHome() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session = request.getSession();
if(session.getAttribute("uname")!=null)
{
response.getWriter().print("Welcome: "+session.getAttribute("uname"));
}
else
{
response.sendRedirect("login.html");
}
}
}
Video: Session Object
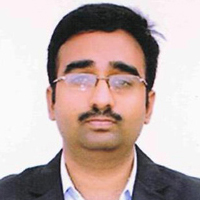
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply