In this article we will look at what is buffer overflow attack and also learn the two types of buffer overflow attacks along with code example.
Contents
What is Buffer Overflow Attack
Buffer overflow or buffer overrun is an anomaly in the code or process which allows an attacker to store data in a buffer outside its own memory and execute malicious code to compromise the security of the system. A buffer is a storage location in the main memory. An array in C and C++ languages
can be considered as a buffer.
Watch the below video to understand about a buffer overflow attack:
ARVE Error: src mismatchprovider: youtube
url: https://www.youtube.com/watch?v=X1P0AXh90Xs&list=PL_RcVnBPGmSLAGyNa6wiAf8bbVwxYYzCi&index=16
src in org: https://www.youtube.com/embed/X1P0AXh90Xs?list=PL_RcVnBPGmSLAGyNa6wiAf8bbVwxYYzCi
src gen org: https://www.youtube.com/embed/X1P0AXh90Xs
In a buffer overflow attack, the attacker might provide malicious input to be stored in the buffer and this overwrites the data in the adjacent memory areas. Every process has an image in the main memory and is illustrated as shown below:
If the program contains functions, the data related to a function is stored on the stack and if the program creates any runtime data, such data is allocated on the heap.
Types of Buffer Overflow
Based on whether stack or heap is exploited, there are two types of buffer overflows:
2. Heap-based buffer overflow
Stack-based Buffer Overflow
A stack-based buffer overflow works by overwriting a buffer stored on the stack. The characteristics of stack-based programming are as follows:
- Stack is a memory space in which automatic variables are allocated
- Function parameters are allocated on the stack
- After the execution of a function is completed, the reference to the variable on the stack is removed
The attacker may exploit stack-based buffer overflows to manipulate the program in various ways by overwriting:
- A local variable that is near the buffer in memory on the stack to change the behavior of the program
- The return address in a stack frame
- A function pointer or exception handler which is subsequently executed
The factors that contribute to overcome the exploits are:
- Null bytes in addresses
- Variability in the location of shellcode
- Differences between environments
Languages like C and C++ are vulnerable to buffer overflow attacks as these languages have no runtime checks whether the process is accessing valid addresses or not. These languages also have no checking against whether the data is stored within the boundaries of the array’s memory or not.
Consider the following program which is written in C language:
#include <stdio.h> #include <string.h> void func(char *name) { char buf[100]; strcpy(buf, name); printf("Welcome %s\n", buf); } int main(int argc, char *argv[]) { func(argv[1]); return 0; }
In the above code, when the program is executed, the function func is allocated memory on the stack. Memory is allocated for the parameter name followed by func’s return address (the address of the line return 0 in main function) and a special base pointer as shown below:
If the above program is executed by typing ./buf Mathilda, the program executes normally and the output will be Welcome Mathilda. Here, buf is the name of the program and Mathilda is a command line argument. Note that the string Mathilda is stored in the array named buf which is allocated on the stack.
Now, an attacker can provide malicious input as shown below:
As the given input is greater than the size of the array (which is 100), the given input starts overwriting the memory locations adjacent to the buffer as shown below:
This way an attacker can write shellcode along with other text as a command line argument. Shellcode is small piece of code which when executed presents the attacker with a shell to execute further commands.
Heap Buffer Overflow
Heap buffer overflow occurs in the heap section of the memory. An attacker might insert crafted input into a buffer which is allocated memory on the heap and cause overflow. A program or process is vulnerable to heap buffer overflow if it doesn’t check for the boundaries where the data is stored. The
characteristics of heap-based programming are:
- Heap is a storage in main memory where dynamic data objects are allocated
- Memory created using functions like new(), malloc(), calloc() is allocated on the heap
- Dynamically created variables or objects are allocated memory on the heap
In heap buffer overflow, the attacker tries to overwrite the internal structures such as linked list pointers. The canonical heap overflow technique overwrites dynamic memory allocation linkage (such as malloc metadata) and uses the resulting pointer exchange to overwrite a program function pointer.
Following measures helps to minimize buffer overflow attacks:
- Assessment of secure code manually
- Disable stack execution
- Compiler tools
- Dynamic run-time checks
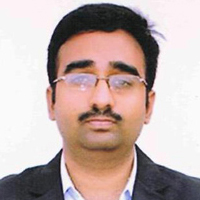
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply