This article provides a comprehensive overview of control statements in Python programming language along with example programs.
Contents
Introduction
Generally, a Python script executes in a sequential manner. If a set of statements should be skipped or repeated again, we should alter the flow of control. The statements which allow us to alter the flow of control are known a control statements. Python supports the following control statements:
- if
- if..else
- elif ladder
- while
- for
- break
- continue
if Statement
A if statement can be used as a one way decision making statement. The syntax of if statement is as follows:
if condition:
statement1
statement2
....
statementN
if…else Statement
A if…else statement can be used as a two way decision making statement. The syntax of if…else statement is as follows:
if condition:
statement1
statement2
....
statementN
else:
statement1
statement2
....
statementN
if…else Ternary Expression
Python supports if…else ternary expression. Its syntax is as follows:
expr1 if condition else expr2
Following is an example which demonstrates if…else ternary expression:
a=10
b=5
c = a if a>b else b
print(c)
while Loop
A while loop can be used to repeat a set of statements based on a condition. The syntax of while loop is as follows:
while test:
statements
else: #optional
statements
As mentioned above, else part is optional. The else part executes only when break is not used.
for Loop
A for loop can be used to repeat a set of statements. The syntax of for loop is as follows:
for target in object:
statements
else: #optional
statements
The object in the above syntax should be a collection of values like a list, string, tuple, etc. Like while loop, the else part is optional and executes only when break is not used.
break and continue
Both break and continue and jump statements which are used inside while loop or for loop. When break is used inside the loop, the control moves to the statement outside the enclosing loop. When continue is used inside the loop, the control moves to the first statement of the enclosing loop skipping all the remaining statements after the continue statement inside the enclosing loop.
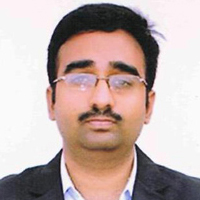
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply