This article provides a comprehensive overview of modules along with examples in Python programming language.
Contents
Introduction
A function allows to reuse a piece of code. A module on the other hand contains multiple functions, variables, and other elements which can be reused. A module is a Python file with .py extension. Each .py file can be treated as a module.
We can print the name of existing module by using the __name__ attribute of the module as follows:
print(“Name of module is:”, __name__)
Above code prints the name of module as __main__. By default the name of the current module will be __main__.
Module Loading and Execution
A module imported in a Python program should available where the program file is located. If the module is not available where the program file is, it looks for the module in the directories available in PYTHONPATH. If the module is still not found, it will search in the lib directory of Python installation. If still not found, it will generated error names ImportError.
Once a module is located, it is loaded into memory. A compiled version of the module will be created with .pyc extension. When the module is referenced next time, the .pyc file will be loaded into memory instead of recompiling it. A new compiled version (.pyc) will be generated whenever it is out of date. Programmer can also force the Python shell to recompile the .py file by using the reload function.
import Statement
The import keyword allows to use functions or variables available in a module. Syntax of import statement is as follows:
import module_name
After importing the module, we can access functions and variables available in it as follows:
module_name.variable_name
module_name.function_name(arguments)
Following program imports the pre-defined sys module and prints the PYTHONPATH information by using the path variable as follows:
import sys
print(sys.path)
Following program imports the pre-defined random module and prints a random number by calling the choice function as follows:
import random
print(random.choice(range(1,101)))
Above code generates a random number in the range 1 to 100.
from…import Statement
A module contains several variables and functions. When we use import statement we are importing everything in the module. To import only selected variables or functions from the module, we use from…import statement. To import everything from the module we can write:
from module-name import *
For example to import the pi variable from the math module we can write:
from math import pi
Now, we can directly use pi variable in our program as:
pi * r * r
We can import multiple elements from the module as:
from module-name import ele1, ele2, ...
We can also import a module element with another name (alias) using the as keyword as follows:
from module-name import element as newname
We can access the command line parameters passed to a Python script using the argv variable available in the sys module as follows:
import sys
print(sys.argv[0], sys.argv[1], ...)
We can terminate a Python script abruptly by using the exit() method as follows:
import sys
sys.exit(“Error”)
Creating Modules
A python file with .py extension is treated as a module. For example, the below script is saved as circle.py:
from math import pi
def area(r):
return pi*r*r*r
def peri(r):
return 2*pi*r
Following is the main module file named test.py in which we import our own module circle as follows:
import circle
r = int(input())
print("Area of circle is:", circle.area(r))
Notice that we are using area() function which was defined in the module circle. Remember that circle.py and test.py files should be at the same location.
Steps for creating and using our own module:
- Define the variables, functions, and other elements that you want and save the file as filename.py.
- The filename will server as the module name.
- Now, create a new file (our main module) in which we can import our module by using import or from…import statement.
- Use the variables or functions in the created module using dot (.) operator.
Namespace
A namespace is a logical collection of names. Namespaces are used to eliminate name collisions. Python does not allow programmers to create multiple identifiers with same name. But, in some case we need identifiers having same name.
For example, consider two modules module1 and module 2 have the same function foo() as follows:
#Module 1
def foo():
print(“Foo in module 1”)
#Module 2
def foo():
print(“Foo in module 2”)
When we import both modules into same program and try to refer foo(), error will be generated:
import module1
import module2
foo()
To avoid the error, we have to use fully qualified name as follows:
module1.foo()
module2.foo()
Each module has its own namespace.
Built-in, Global, Local Namespaces
The built-in namespace contains all the built-in functions and constants from the __builtin__ library. The global namespace contains all the elements in the current module. The local namespace contains all the elements in the current function or block. Runtime will search for the identifier first in the local, then in the global, then finally in the built-in namespace.
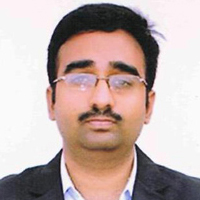
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply