This article provides a comprehensive overview of object oriented programming in Python programming language along with example programs.
Contents
- 1 Introduction to Classes and Objects
- 2 Creating or Defining a Class
- 3 Creating Objects
- 4 Class Variables and Instance Variables
- 5 Public and Private Instance Variables
- 6 self Argument
- 7 __init__() Method (Constructor)
- 8 __del__() Method (Destructor)
- 9 Other Special Methods
- 10 Private Methods
- 11 Built-in Functions
- 12 Built-in Class Attributes
- 13 Garbage Collection
- 14 Class Methods
- 15 Static Methods
Introduction to Classes and Objects
Classes and objects are the two basic concepts in object oriented programming. An object is an instance of a class. A class creates a new type and provides a blueprint or a template using for creating objects. In Python we already know that everything is an object of some class. In Python, the base class for all classes is object.
Creating or Defining a Class
The syntax for a class is as follows:
class class_name:
<statement_1>
<statement_2>
...
...
<statement_n>
The statements in the syntax can be variables, control statements, or function definitions. The variables in a class are known as class variables. The functions defined in a class are known as class methods. Class variables and class methods are together called as class members.
The class methods can access all class variables. The class members can be accessed outside the class by using the object of that class. A class definition creates a new namespace.
Creating Objects
Once a class is defined, we can create objects for it. Syntax for creating an object is as follows:
object_name = class_name()
Creating an object for a class is known as instantiation. We can access the class members using an object along with the dot (.) operator as follows:
object_name.variable_name
object_name.method_name(args_list)
Following code creates a student class and one object s which is used to access the members of student class:
class Student:
#branch is a class variable
branch = "CSE"
def read_student_details(self, rno, n):
#regdno and name are instance variables
self.regdno = rno
self.name = n
def print_student_details(self):
print("Student regd.no. is", self.regdno)
print("Student name is", self.name)
print("Student branch is", Student.branch)
s = Student( ) #s is an object of class Student
s.read_student_details("16PA1A0501", "Ramesh")
s.print_student_details()
Class Variables and Instance Variables
Variables which are created inside a class and outside methods are known as class variables. Class variables are common for all objects. So, all objects share the same value for each class variable. Class variables are accessed as ClassName.variable_name.
Variables created inside the class methods and accessed using the self variable are known as instance variables. Instance variable value is unique to each object. So, the values of instance variables differ from one object to the other. Consider the following class:
class Student:
#branch is a class variable
branch = "CSE"
def read_student_details(self, rno, n):
#regdno and name are instance variables
self.regdno = rno
self.name = n
def print_student_details(self):
print("Student regd.no. is", self.regdno)
print("Student name is", self.name)
print("Student branch is", Student.branch)
In the above Student class, branch is a class variable and regdno and name are instance variables.
Public and Private Instance Variables
By default instance variables are public. They are accessible throughout the class and also outside the class. An instance variable can be made private by using _ _ (double underscore) before the variable name. Private instance variables can be accessed only inside the class and not outside the class. Following example demonstrates private instance variable:
class Student:
#branch is a class variable
branch = "CSE"
def read_student_details(self, rno, n):
#regdno and name are instance variables
self.__regdno = rno #regdno is private variable
self.name = n
def print_student_details(self):
print("Student regd.no. is", self.__regdno)
print("Student name is", self.name)
print("Student branch is", Student.branch)
s = Student()
s.read_student_details("16PA1A0501", "Ramesh")
s.print_student_details()
print(s.__regdno) #This line gives error as ___regdno is private.
self Argument
The self argument is used to refer the current object (like this keyword in C and Java). Generally, all class methods by default should have at least one argument which should be the self argument. The self variable or argument is used to access the instance variables or object variables of an object.
__init__() Method (Constructor)
The __init__() is a special method inside a class. It serves as the constructor. It is automatically executed when an object of the class is created. This method is used generally to initialize the instance variables, although any code can be written inside it.
Syntax of __init__() method is as follows:
def __init__(self, arg1, arg2, ...):
statement1
statement2
...
statementN
For our Student class, the __init__() method will be as follows:
def __init__(self, rno, n):
#regdno and name are instance variables
self.regdno = rno
self.name = n
__del__() Method (Destructor)
The __del__() method is a special method inside a class. It serves as the destructor. It is executed automatically when an object is going to be destroyed. We can explicitly make the __del__() method to execute by deleting an object using del keyword.
Syntax of __del__() method is as follows:
def __del__(self):
statement1
statement2
...
statementN
For our Student class, __del__() method can be used as follows:
def __del__(self):
print("Student object is being destroyed");
Other Special Methods
Following are some of the special methods available in Python:
Method | Description |
__repr__() | This method returns the string representation of an object. It works on any object, not just user class instances. It can be called as repr(object). |
__cmp__() | This method can be used to override the behavior of == operator for comparing objects of a class. We can also write our own comparison logic. |
__len__() | This method is used to retrieve the length of an object. It can be called as len(object). |
__call__() | This method allows us to make a class behave like a function. Its instance can be called as object(arg1,arg2,…) |
__hash__() | While working with sets and dictionaries in Python, the objects are stored based on hash value. This value can be generated using this method. |
__lt__() | This method overrides the behavior of < operator. |
__le__() | This method overrides the behavior of <= operator. |
__eq__() | This method overrides the behavior of = operator. |
__ne__() | This method overrides the behavior of != operator. |
__gt__() | This method overrides the behavior of > operator. |
__ge__() | This method overrides the behavior of >= operator. |
__iter__() | This method can be used to override the behavior of iterating over objects. |
__getitem__() | This method is used for indexing, i.e., for accessing a value at a specified index. |
__setitem__() | This method is used to assign an item at a specified index. |
Private Methods
Like private variables, we can also created private methods. When there is a need to hide the internal implementation of a class, we can mark the method as private.
It is not recommended to access a private method outside the class. But if needed, we can access a private method outside the class as follows:
objectname._classname__privatemethod(arg1, arg2, ...)
If needed, we can access private variables using similar syntax as above.
Following example demonstrates a private method:
class Box:
def __init__(self, l, b):
self.length = l
self.breadth = b
#Private method
def __printdetails(self):
print("Length of box is:", self.length)
print("Breadth of box is:", self.breadth)
def display(self):
self.__printdetails()
b = Box(10,20)
b. display() #recommended
b._Box__printdetails() #not recommended
Built-in Functions
Following are some of the built-in functions to work with objects:
Function | Description |
hasattr(obj, name) | This function checks whether the specified attribute belongs to the object or not. |
getattr(obj, name[,default]) | This function retrieves the value of the attribute on an object. If the default value is given it returns the that if no attribute is present. Otherwise, this function raises an exception. |
setattr(obj, name, value) | This function is used to set the value of an attribute on a given object. |
delattr(obj, name) | This function deletes an attribute on the given object. |
Built-in Class Attributes
Every class in Python by default has some attributes. Those attributes can be accessed using dot (.) operator. These attributes are as follows:
Attribute | Description |
__dict__ | Gives the dictionary containing the namespace of a class. |
__doc__ | Gives the documentation string of the class if specified. Otherwise, it returns None. |
__name__ | Gives the name of the class |
__module__ | Gives the name of the module in which the class is defined |
__bases__ | Gives the base classes in inheritance as a tuple. Otherwise, it returns an empty tuple. |
Garbage Collection
Python runs a process in the background which frees an object from memory when it is no longer needed or if it goes out of scope. This process is known as garbage collection. Python maintains a reference count for each object in the memory.
The reference count increases when we create aliases or when we assign an object a new name or place it in a list or other data structure. Reference count decreases when an object goes out of scope and it becomes zero when the object is deleted using del keyword.
Class Methods
A class method is a method which is marked with classmethod decorator and the first argument to the method is cls. A class method is called using the class name. Class methods are generally used as a factory method to create instances of the class with the specified arguments. Example of a class method is as follows:
class Box:
def __init__(self, l, b):
self.length = l
self.breadth = b
def display(self):
print("Length of box is:", self.length)
print("Breadth of box is:", self.breadth)
@classmethod
def CreateInstance(cls, l, b):
return cls(l, b)
b = Box.CreateInstance(10,20)
b. display()
Static Methods
A static method is a method marked with staticmethod decorator. Code that manipulates information or data of a class is placed in a static method. Static method does not depend on the state of the object. Static method can be called using class name. Static method does not have any additional arguments like self and cls.
Following example demonstrates a static method:
class Box:
sides = None
def __init__(self, l, b):
self.length = l
self.breadth = b
def display(self):
print("Length of box is:", self.length)
print("Breadth of box is:", self.breadth)
@staticmethod
def init_sides(s):
sides = s
print("Sides of box =", sides)
Box.init_sides(4)
b = Box(10,20)
b. display()
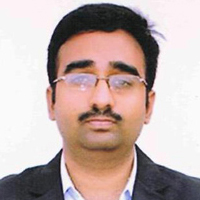
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply