This article provides a comprehensive introduction to object oriented paradigm along with real-world examples.
This world is made up off several objects and objects communicate with each other. Similar objects can be grouped together. For example, take a college. It can have two working sections like teaching and non-teaching. These two groups can be further sub divided.
We can also group the people in a college into several departments. Generally a college will have several lecturers in each department. A college can have one or more laboratories and there might be separate lab technicians to assist in conducting practical. A college will have a Principal for managing the entire college. Each department might have its own head who is responsible for managing their own department. Like this the entire world can be viewed as a collection of objects that communicate with each other to perform activities.
This theory of objects can be applied to software to solve complex problems.
Contents
Disadvantages of Conventional Programming
Traditional programming languages like COBOL, FORTRAN etc are known as procedural languages. In these languages the program is written as a set of statements which contains the logic and control that instructs the compiler or interpreter how to perform a certain task. It becomes difficult to manage the code when the program is quite large.
To reduce complexity, functions or modules were introduced which divides a large program into a set of cohesive units. Each unit is called a function and each function can call other functions as shown below:
Even though functions reduces complexity, with a lot of functions sharing a common set of global data may lead to logical errors.
Following are the disadvantages of procedural or structured languages:
- Programs are divided into a set of functions which can share data. Hence, no data security.
- Focus is on the code to perform the task but not on the data needed.
- Data is shared globally by several functions which might lead to logical errors.
- No restrictions on which functions can share the global data.
Concepts of Object-Oriented Programming
Following are the key concepts in object-oriented programming:
Objects
Object is a real world entity or a prime run-time entity in object-oriented programming. Object occupies space in memory. Every object has its own properties and behavior. An object is an instance or specimen of a class. Every object is unique. Each object contains state, which is the collection of data values associated with the properties of an object.
Some of the real world examples for objects are shown below:
Classes
A class is a collection of similar objects that have similar properties and exhibit similar behavior. A class is a template or blueprint for creating objects. After a class is created, we can create any number of objects. A class does not occupy any memory like an object.
Real world examples of classes are shown below:
Methods
An operation represents the behavior of an object. When an operation is coded in a class, it is called a method. All objects in a class perform certain common actions or operations. Following figure shows a class, its data members, and methods written in different styles:
Data Abstraction
Abstraction refers to the process of presenting essential features without including any complex background details. A class uses this abstraction to represent only essential properties and behavior.
A powerful way to achieve abstraction is by manipulating hierarchical classifications. This allows us to divide the system into several logical layers which can be maintained separately.
Real world example for abstraction is a computer which is a single unit made up of several units (parts) as shown below:
Encapsulation
Wrapping up of code and data together into a single unit is known as encapsulation. Encapsulation is implemented by classes. A class contains data which is generally private and is accessible to the outside world only through public methods.
A side effect of encapsulation is data hiding which allows the users to use an object without knowing its internal details. Following figure represents different sections of encapsulation:
Inheritance
Deriving properties of an object from one class to object of an another class is known as inheritance. Inheritance promotes the feature of object-oriented programming, reusability.
Using inheritance a new class can be created without effecting the existing class. We can use already available functionality of the existing class and add new functionality in the new class based on the requirements.
Polymorphism
Single method exhibiting different behaviours in the same class or different classes is known as polymorphism. We can code a generic interface (set of methods) to a set of associated actions.
As a real world example for polymorphism consider a generic Line class which contains a method Display(). Now consider three specific classes: DottedObject, SingleObject, and DashObject which in turn also contains the same Display() method as shown below:
Dynamic Binding
The process of associating an action to a message at run-time is known as dynamic binding which is also known as late binding. The action to be associated is recognized when the object is created which is done at run-time.
As an example, consider the above hierarchy in which a Line object can call the Display() method in any of the three child classes: DottedObject, SingleObject, or DashObject.
Message Passing
Objects communicate through messages. A message is a request for an action. Object sending the message can also pass data (parameters). Object which receives the message invokes a corresponding member function (action).
Consider the following example which represents message passing:
Reusability
Using the code available in an existing class to create new classes is known as reusability which is an important feature of object-orientation. In the below example, class A is an already existing class from which class B is created and from class B, class C is created:
Delegation
In object-oriented programming, two classes can be joined either by inheritance or delegation. Both of them promotes reusability of code.
As shown in the below figure (a), class Y is derived from class X. Here class Y is a kind of X. This kind of relationship between Y and X classes is known as inheritance. Inheritance is also known as is a relationship.
Also as shown in figure (b) below, objects of classes A and B are members of class C. This kind of relationship is known as has a relationship and is also called as delegation or composition.
Genericity
The software components (like classes) can have more than one implementation based on the data type of arguments. Programmer can create a generic class or function which can work with different types of arguments. The template feature in C++ allows generic programming.
For example, a list data structure can operate on integers, characters, strings, floats or any other types of objects. So, a programmer can create a generic List class which can accept any kind of data.
Advantages of OOP
Object-oriented programming offers many advantages to the programmers and users. This technology allows programmers to create high quality software at an affordable price. Following are the advantages of OOP:
- Object-oriented programs can be upgraded easily.
- Using inheritance, code redundancy can be eliminated.
- Data hiding allows the programmers to develop safe programs that do not disturb code in other parts of the program.
- Encapsulation allows programmers to create classes with several features and only few public methods to be exposed.
- All object-oriented languages can create, extend and reuse existing programs.
- Object-oriented programming enhances the thought process of a programmer leading to rapid development of software.
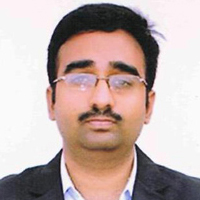
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply