This article provides a comprehensive overview of strings in Python programming language. All the string functions are demonstrated with examples.
Contents
Introduction to Strings in Python
A string is a sequence of one character strings. Strings are used to store textual information. Examples of strings:
‘Python’
‘Ramesh Kumar’
“A”
“123”
Sequence Operations on Strings
We can find the length (number of characters) of a string using the pre-defined function len:
>>>s = “hello”
>>>len(s)
5
We can also fetch individual characters from the strings using indexing. The index of first character starts from 0, next is 1, and so on:
>>>s = “hello”
>>>s[0]
‘h’
Negative indexes are used to index from right to left in a string:
>>>s = “Python”
>>>s[-1]
‘n’
Sequences like strings also support another form of indexing called slicing which is used to extract a part of the string:
>>>s = “Python”
>>>s[1:3]
‘yt’
More examples on slicing:
>>>s = “Python”
>>>s[2:]
thon
>>>s[:4]
‘Pyth’
>>>s[:len(s)]
‘Python’
>>>s[:-1]
‘Pytho’ #Everything except last character
>>>s[:]
‘Python’
Strings can be concatenated using the ‘+’ operators as shown below:
>>>s = “Python”
>>>s + “ Rocks”
‘Python Rocks’
A string can be printed multiple times by using the power (**) operator as shown below:
>>>s = “Yo ”
>>>s**5
‘Yo Yo Yo Yo Yo ’
String Immutability
In Python, every object can be classified as mutable or immutable. Numbers, strings, and tuples are immutable, i.e., once assigned, values cannot be changed. Lists, dictionaries, and sets are mutable. For example, following will give error:
>>>s = “Python”
>>>s[1] = ‘a’
...
TypeError: 'str' object does not support item assignment
String Specific Methods
The find method can be used to find position of a given substring in the string:
>>>s=“Python”
>>>s.find(‘Py’)
0
>>>s.find(‘ab’)
-1
The replace method can be used to replace a substring with a given string:
>>>s=“Python”
>>>s.replace(‘Py’, ‘Go’)
‘Gothon’
>>>s.replace(‘ab’, ‘Go’)
‘Python’
The split method can be used to split a line into words based on the given delimiter. Default delimiter is space:
>>>line='this is a line‘
>>>line.split()
['this', 'is', 'a', 'line']
>>>line=‘this,is,a,line’
>>>line.split(‘,’)
['this', 'is', 'a', 'line']
The upper and lower methods can be used to turn a string into upper or lower case respectively:
>>>s=‘awesome’
>>>s=s.upper()
>>>s
‘AWESOME’
>>>s=s.lower()
>>>s
‘awesome’
The isalpha and isdigit methods can be used to find whether a string is containing all alphabets or digits respectively:
>>> s='alpha'
>>> s.isalpha()
True
>>> s.isdigit()
False
>>> s='123'
>>> s.isdigit()
True
The rstrip method can be used to remove white spaces at the right end of the string:
>>> line='this is a line '
>>> line.rstrip()
'this is a line'
>>> line
'this is a line '
We can substitute values in a string as follows:
>>> lang='Python'
>>> ver='3.x'
>>> 'Welcome to %s. Version %s.' % (lang,ver)
'Welcome to Python. Version 3.x.'
>>> 'Welcome to {0}. Version {1}.'.format(lang,ver)
'Welcome to Python. Version 3.x.'
>>> 'Welcome to {}. Version {}.'.format(lang,ver)
'Welcome to Python. Version 3.x.'
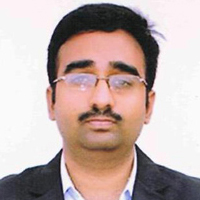
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply