Proxy pattern is used to provide a surrogate or placeholder for another object to control access to it. Let’s learn in detail about this design pattern.
Contents
- 1 Proxy Pattern’s Intent
- 2 Also Known As
- 3 Proxy Pattern’s Motivation
- 4 Proxy Pattern’s Applicability
- 5 Proxy Pattern’s Structure
- 6 Participants
- 7 Collaborations
- 8 Proxy Pattern’s Consequences
- 9 Proxy Pattern’s Implementation
- 10 Sample Code (Java Example)
- 11 Proxy Pattern’s Known Uses
- 12 Related Patterns
- 13 Non-Software Example
Proxy Pattern’s Intent
To provide a surrogate or placeholder for another object to control access to it.
Also Known As
Surrogate
Proxy Pattern’s Motivation
Sometimes we need the ability to control the access to an object. For example if we need to use only a few methods of some costly objects we’ll initialize those objects when we need them entirely. Until that point we can use some light objects exposing the same interface as the heavy objects.
These light objects are called proxies and they will instantiate those heavy objects when they are really need and by then we’ll use some light objects instead.
This ability to control the access to an object can be required for a variety of reasons: controlling when a costly object needs to be instantiated and initialized, giving different access rights to an object, as well as providing a sophisticated means of accessing and referencing objects running in other processes, on other machines.
Consider for example an image viewer program. An image viewer program must be able to list and display high resolution photo objects that are in a folder, but how often do someone open a folder and view all the images inside.
Sometimes you will be looking for a particular photo, sometimes you will only want to see an image name. The image viewer must be able to list all photo objects, but the photo objects must not be loaded into memory until they are required to be rendered.
Proxy Pattern’s Applicability
Proxy pattern is applicable when:
- A remote proxy provides a local representative for an object in a different address space.
- A virtual proxy creates expensive objects on demand.
- A protection proxy controls access to the original object. Protection proxies are useful when objects should have different access rights.
- A smart reference is a replacement for a bare pointer that performs additional actions when an object is accessed.
Proxy Pattern’s Structure
The structure of proxy pattern is as shown below:
Participants
The participants in proxy pattern are:
- Proxy: Maintains a reference that lets the proxy access the real subject. Provides an interface identical to Subject so that the Proxy can be substituted for the real subject. Controls access to the real subject.
- Subject: Defines the common interface for RealSubject and Proxy so that a Proxy can be used anywhere a RealSubject is expected.
- RealSubject: Defines the real object that the proxy represents.
Collaborations
Proxy forwards requests to RealSubject when appropriate, depending on the kind of proxy.
Proxy Pattern’s Consequences
The Proxy pattern introduces a level of indirection when accessing an object. The additional indirection has many uses, depending on the kind of proxy:
- A remote proxy can hide the fact that an object resides in a different address space.
- A virtual proxy can perform optimizations such as creating an object on demand.
- Both protection proxies and smart references allow additional housekeeping tasks when an object is accessed.
Proxy Pattern’s Implementation
The Proxy pattern can exploit the following language features:
- Overloading the member access operator in C++: C++ supports overloading operator->, the member access operator. Overloading this operator lets you perform additional work whenever an object is dereferenced.
- Using doesNotUnderstand in Smalltalk: Smalltalk provides a hook that you can use to support automatic forwarding of requests. Smalltalk calls doesNotUnderstand: aMessage when a client sends a message to a receiver that has no corresponding method. The Proxy class can redefine doesNotUnderstand so that the message is forwarded to its subject.
- Proxy doesn’t always have to know the type of real subject: If a Proxy class can deal with its subject solely through an abstract interface, then there’s no need to make a Proxy class for each RealSubject class; the proxy can deal with all RealSubject classes uniformly. But if Proxies are going to instantiate RealSubjects (such as in a virtual proxy), then they have to know the concrete class.
Sample Code (Java Example)
Let’s say we have a class that can run some command on the system. Now if we are using it, its fine but if we want to give this program to a client application, it can have severe issues because client program can issue command to delete some system files or change some settings that you don’t want. Here a proxy class can be created to provide controlled access of the program.
Since we code Java in terms of interfaces, here is our interface and its implementation class:
//CommandExecutor.java
public interface CommandExecutor {
public void runCommand(String cmd) throws Exception;
}
//CommandExecutorImpl.java
import java.io.IOException;
public class CommandExecutorImpl implements CommandExecutor {
@Override
public void runCommand(String cmd) throws IOException {
//some heavy implementation
Runtime.getRuntime().exec(cmd);
System.out.println("'" + cmd + "' command executed.");
}
}
Now we want to provide only admin users to have full access of above class, if the user is not admin then only limited commands will be allowed. Here is our very simple proxy class implementation:
//CommandExecutorProxy.java
public class CommandExecutorProxy implements CommandExecutor {
private boolean isAdmin;
private CommandExecutor executor;
public CommandExecutorProxy(String user, String pwd){
if("Pankaj".equals(user) && "J@urnalD$v".equals(pwd)) isAdmin=true;
executor = new CommandExecutorImpl();
}
@Override
public void runCommand(String cmd) throws Exception {
if(isAdmin){
executor.runCommand(cmd);
}else{
if(cmd.trim().startsWith("rm")){
throw new Exception("rm command is not allowed for non-admin users.");
}else{
executor.runCommand(cmd);
}
}
}
}
Here is the client program::
//ProxyPatternTest.java
public class ProxyPatternTest {
public static void main(String[] args){
CommandExecutor executor = new CommandExecutorProxy("Pankaj", "wrong_pwd");
try {
executor.runCommand("ls -ltr");
executor.runCommand(" rm -rf abc.pdf");
} catch (Exception e) {
System.out.println("Exception Message::"+e.getMessage());
}
}
}
Proxy Pattern’s Known Uses
Following are the examples in Java API where proxy pattern is used:
- lang.reflect.Proxy
- rmi.*, the whole API actually.
Related Patterns
An adapter provides a different interface to the object it adapts. In contrast, a proxy provides the same interface as its subject. However, a proxy used for access protection might refuse to perform an operation that the subject will perform, so its interface may be effectively a subset of the subject’s.
Although decorators can have similar implementations as proxies, decorators have a different purpose. A decorator adds one or more responsibilities to an object, whereas a proxy controls access to an object.
Non-Software Example
The Proxy provides a surrogate or place holder to provide access to an object. A check or bank draft is a proxy for funds in an account. A check can be used in place of cash for making purchases and ultimately controls access to cash in the issuer’s account.
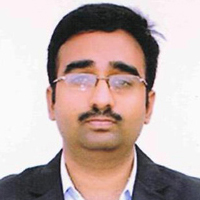
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply