This article provides a comprehensive overview of input and output in C++ programming language along with example programs.
Any program in a given programming language needs significant amount of input from the user and also needs to display to the output several times.
To support such I/O operations, C++ provides a library which contains several classes and functions.
Contents
Streams in C++
In C++, standard library maintains input and output as streams.A stream is a flow of data, measured in bytes, in sequence.
If data is received from input devices, it is called a source stream and if the data is to be sent to output devices, it is called a destination stream.
The data in the source stream can be used as input to the program. Then it is called an input stream.
The destination stream can be used by the program to send data to the output devices. Then it is called an output stream.
Pre-Defined Streams
C++ contains number of pre-defined streams. They are also called as standard I/O objects.
These streams are available automatically when the execution of a program starts.
The pre-defined streams available in C++ are:
- cin: Standard input, usually keyboard. It handles input devices. It is analogous to stdin in C.
- cout: Standard output, usually monitor. It handles output devices. It is analogous to stdout in C.
- cerr: Standard error output, usually screen. It handles the unbuffered output data. It is analogous to stderr in C.
- clog: A fully buffered version of cerr. It handles error messages that are sent from buffer to the standard error device. There is no equivalent of clog in C.
Stream Classes
C++ provides several classes to work with stream based I/O.
Such classes are known as stream classes and they are arranged in a hierarchy shown below:
All these classes are declared in iostream.h header file.
So, we have to include this header file in order to use the stream classes.
As shown in the above figure, ios is the base class for all the stream classes from which istream and ostream classes are derived.
The iostream class is derived from both istream and ostream classes.
Following table lists out some of the stream classes and their contents:
Formatted and Unformatted Data
Data which is received by the program without any modifications and sent to the output device without any modifications is known as unformatted data.
On the other hand, sometimes we may want to apply some modifications to the actual data that is being received or sent.
For example, we might want to display an integer in hexadecimal format in the output, leave some white space when printing a number and adjustments in the decimal point.
Such modified data in known as formatted data.
As an example for formatted data, if we want to display a decimal number in hexadecimal format, we can use the hex manipulator as shown below:
cout<<hex<<15
Above line displays 15 in hexadecimal format as F.
Unformatted Console I/O
The input stream uses cin object of istream class to read data and the output stream uses cout object of ostream class to display data on output devices.
The cin statement uses >> (extraction operator) to read data from keyboard.
Syntax of cin statement is shown below:
cin>>variable-name;
Example:
int a;
float f;
char ch;
cin>>a>>f>>ch;
In the above example, cin is used in cascading fashion.
This is helpful for reading values into multiple variables in a single statement.
No need of any format specifiers like %d, %f etc.
The >> (extraction) operator will take care of that automatically.
If the data provided is greater than the number of variables in the cin statement, extra data will remain in the input stream.
The cout statement uses << (insertion operator) to display data to the output device.
Syntax of cout statement is shown below:
cin>>variable-name;
Example:
cout<<a<<b<<c;
In the above example, a, b, and c are variables.
No need to specify format specifiers like %d, %f, etc.
The << (insertion) operator will take care of that automatically.
All escape sequences like “\n”, “\t”, etc, can be used in cout statement.
Example:
//C++ program to read two numbers and print their sum
#include <iostream>
using namespace std;
int main()
{
int a, b;
cin>>a>>b; //Reading values into variables a and b
cout<<"Sum is: "<<(a+b); //Printing the sum
return 0;
}
Output of the above program is:
Sum is: 30
To read a single character, we can use get() function of istream class and to print a single character, we can use put() function of ostream class.
Syntax of these methods is as follows:
get(char)
get(void)
put(char)
Example:
//C++ program to demonstrate get() and put() functions
#include <iostream>
using namespace std;
int main()
{
char ch1,ch2,ch3;
cin.get(ch1).get(ch2).get(ch3);
cout.put(ch1).put(ch2).put(ch3);
return 0;
}
Input: xyz
Output of the above program is: xyz
To read a string or a line of text, we can use getline() function and to print a string we can use write() function.
Syntax of both of these methods is as follows:
getline(string, size)
write(string, size)
The size in the above syntax specifies the number of characters to read and print in the given string.
Example:
//C++ program to demonstrate getline() and write() functions
#include <iostream>
using namespace std;
int main()
{
char str[30];
cin.getline(str, 10);
cout.write(str, 10);
return 0;
}
Input: C++ rocks
Output of the above program is:
C++ rocks
istream Class Functions
The istream class is derived from ios class.The istream class contains the following functions:
Formatted Console I/O
C++ provides various console I/O functions for formatted input and output.
The three ways to display formatted input and output are:
- ios class functions and flags
- Standard manipulators
- User-defined manipulators
ios Class
The ios class is the base class for all input and output classes in C++.Following are some of the functions available in ios class:
The width() function can be used in two ways as shown below:
- int width() : Returns the current width setting.
- int width(int) : Sets the specified width and returns the previous width setting.
The precision() function can be used in two ways as shown below:
- int precision() : Returns the current precision setting.
- int precision(int) : Sets the specified precision and returns the previous precision setting..
The fill() function can be used in two ways as shown below:
- char fill() : Returns the current fill character.
- char fill(char) : Sets the fill character and returns the previous fill character.
The setf() function can be used in two ways as shown below:
- long setf(long sb, long f) : The bits specified by the variable f are removed from data member x_flags in ios and set according the value specified by sb.
- long setf(long) : Sets the flags in x_flags based on the given value.
Bit Fields
The ios class contains several flags and bit fields to adjust or format the output displayed on the screen.
Available bit fields and flags in ios class are as follows:
ios::adjustfield is used with setf() function to set the alignment of padding to left, right, or internal.
ios::floatfield is used with setf() function to set the floating point notation to scientific or fixed.
ios::basefield is used with setf() function to set the display notation to decimal, octal or hexadecimal.
Flags without Bit Fields
Some flags does not contain any bit fields. Such flags are listed below:
Note: In the above table replace Ios with ios (sorry for the typo).
Manipulators
Manipulators are helper functions which can be used for formatting input and output data.The ios class and iomanip.h header file has several pre-defined manipulators.
Below table lists out several pre-defined manipulators.
The manipulators hex, oct, dec, ws, endl, and flush are defined in iostream.h.
The manipulators setbase(), width(), fill(), etc., that require an argument are defined in iomanip.h.
User-Defined Manipulators
Sometimes, to satisfy custom requirements, we have to create our own manipulator.
Such manipulators which are created by the programmer or user are known as user-defined manipulators.
Syntax for creating a user-defined manipulator is as follows:
ostream & m_name(ostream &os)
{
statement(s);
return os;
}
In the above syntax, m_name is our user-defined manipulator name.
As an example for user-defined manipulator let’s create a manipulator which prints a new line.
Example:
ostream & newl(ostream & os)
{
os<<"\n";
return os;
}
After creating the manipulator named newl, we can use it our program as shown below:
int main()
{
cout<<"Hello"<<newl<<"World"<<newl;
return 0;
}
In the next articles we will learn various basic concepts in C++.
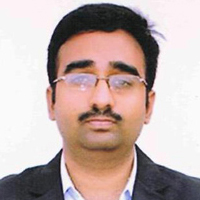
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply