In this article we will look at different data types in C language along with examples. We will learn about three categories of data types.
Visit our C Programming tutorial to learn more about C language.
A data type specifies the type of value that we use in our programs. A data type is generally specified when declaring variables, arrays, functions etc.
In ANSI C, the data types are divided into three categories. They are:
1) Primitive or Fundamental data types
2) User-defined data types
3) Derived data types
Watch this video for explanation of data types in C language:
Contents
Primitive or Fundamental data types
The primitive data types in ANSI C are as shown in the below diagram:
Other compilers support the extended versions of these fundamental data types like: short int, long int, long double etc.
The signed data types are used for storing both positive and negative values where as the unsigned data types are used to store only positive values.
The memory capacity of these data types are based on the hardware.
Watch this video to learn about the size and range of each data type in C language:
The memory capacity of all the fundamental data types in C on a 16-bit machine is as shown below:
User-Defined data types
ANSI C allows the users to define identifiers as their own data types, based on the already existing primitive or fundamental data types.
This concept is known as “type definition” and the data types thus created are known as user-defined data types.
We can create user-defined data types in two ways:
1) By using the “typedef” keyword
2) By using the “enum” keyword
typedef Keyword
The “typedef” keyword can be used to declare an identifier as a user-defined data type.
The syntax for using typedef is as shown below:
typedef int rollno;
typedef float average;
In the above example, rollno and average are new user-defined data types. Now we can use rollno and average as data types as shown below:
rollno r1, r2;
average a1, a2;
enum Keyword
The “enum” is used to declare identifiers as user-defined data types. Such data types are also called as enumerations.
The “enum” keyword can be used to declare an identifier as data type which can store a limited set of integer values.
The syntax of using “enum” keyword is as shown below:
enum identifier {value1, value2, ..., value n};
Example usage of enum is as shown below:
enum days{Mon, Tue, Wed, Thu, Fri, Sat};
In the above example, “days” is a new user-defined type.
All the variables created using the “days” data type can only contain values in the range Mon to Sat as shown in the above example.
We can declare variables by using the “days” data type as shown below:
enum days day1 = Mon;
If we display “day1” in the printf() statement, it will display it as zero.
Generally, the value of a first element in an enumeration always starts with zero and the next element’s value is previous element’s value plus 1.
So, the value of “Tue” will be one and so on.
Derived data types
The data types which are created using the already existing primitive or fundamental types are known as derived data types.
Like user-defined data types we cannot declare new variables using the derived data types.
Examples of derived data types in C are: arrays, functions, structures, unions and pointers.
Let’s learn how to create constants in C in the next article.
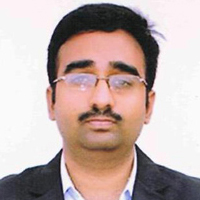
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Sir what’s the syntax for derived datatypes?
Derived data types are arrays, functions, pointers, etc. There are detailed articles for them in this site. You can refer those articles.
Sir may I know the difference between enum and typedef
enum is used for creating new types that have only a restricted set of values.
eg: days (Monday to Sunday), months (1 to 12), gender (M, F) etc.
typedef is for creating new types based on existing types mostly for programmer convenience.
Sir I have a small doubt in long double
The range in long double is 3.4E-4932to1.1E4932
Sir is it 1.1 or 3.4
It is 1.1 only. Not 3.4
Sir could you please give me examples for data types
They are mentioned in the article. Fundamental types like int, char, float, and double. Derived types like: arrays, pointers, structures, and unions etc.
thank you sir